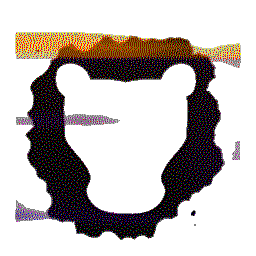 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
72 virtual std::string
alias()
const = 0;
77 virtual void alias(
const std::string &) = 0;
103 virtual std::vector<Tools::ScheduleMappingLWPtr>
virtual const Auth::ValidityInfo & validity() const =0
Retrieve validity status from the credential.
virtual std::vector< Tools::ScheduleMappingLWPtr > lazy_schedules_mapping() const =0
Retrieve the lazy_weak_ptr to ScheduleMapping that map this credential.
virtual Auth::UserId owner_id() const =0
Returns the id of the owner, or 0 if there is no owner (or the owner has no id).
virtual std::string description() const =0
An optional description / notes for the credential.
virtual Auth::UserLPtr owner() const =0
Retrieve the owner of the credential.
This is the header file for a generated source file, GitSHA1.cpp.
odb::lazy_shared_ptr< User > UserLPtr
Base interface for credential objects.
unsigned long CredentialId
virtual std::string alias() const =0
An alias for the credential.
virtual CredentialId id() const =0
Retrieve the identifier of the credential.
virtual size_t odb_version() const =0
Credentials are "optimistic" object (wrt ODB).
A simple class that stores (and can be queried for) the validity of some objects.