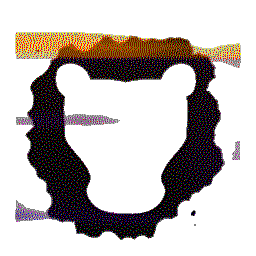 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
37 if (tf.is_in_timeframe(tp))
92 map->schedule_ = assert_cast<SchedulePtr>(shared_from_this());
113 if (name.size() < 3 || name.size() > 50)
115 throw ModelException(
"data/attributes/name",
"Length must be >=3 and <=50.");
117 for (
const auto &c : name)
119 if (!isalnum(c) && (c !=
'_' && c !=
'-' && c !=
'.'))
122 "data/attributes/name",
123 BUILD_STR(
"Usage of unauthorized character: " << c));
#define BUILD_STR(param)
Internal macro.
#define ASSERT_LOG(cond, msg)
An exception class for general API error.