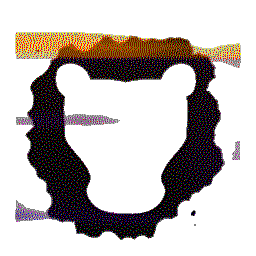 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
30 std::chrono::milliseconds delay)
32 , read_card_strategy_(std::move(read_card))
33 , read_pin_strategy_(std::move(read_pin))
43 using namespace std::chrono;
52 DEBUG(
"Switch to PIN. Current card id = "
63 DEBUG(
"Too slow to enter pin code. Aborting.");
std::unique_ptr< CardReading > CardReadingUPtr
virtual bool completed() const override
Did the strategy gather needed data? If this function returns true, that means that the strategy impl...
void reset() override
Reset self.
An implementation class that represents a Wiegand Reader.
virtual void signal(zmqpp::socket &sock) override
Tells the strategy implementation to send a message to the application containing the received creden...
PinReadingUPtr read_pin_strategy_
@ WIEGAND_CARD_PIN
When reading both a card an a PIN code.
virtual void set_reader(WiegandReaderImpl *new_ptr)
Update the pointer that points back to the associated reader.
CardReadingUPtr read_card_strategy_
void read_reset()
Reset the "read state" of the reader, effectively cleaning the wiegand-bit-buffer and resetting the c...
WiegandCardAndPin(WiegandReaderImpl *reader, CardReadingUPtr read_card, PinReadingUPtr read_pin, std::chrono::milliseconds delay)
Create a strategy that read card and PIN code.
int counter() const
Returns the number of bits read.
virtual void set_reader(WiegandReaderImpl *new_ptr) override
Update the pointer that points back to the associated reader.
The multiple modes available to wiegand reader are implemented through the strategy pattern.
std::chrono::milliseconds delay_
std::unique_ptr< PinReading > PinReadingUPtr
virtual void timeout() override
This is called when the module detect a timeout.
TimePoint time_card_read_
Provide support for Wiegand devices.
WiegandReaderImpl * reader_
const std::string & name() const
Returns the name of this reader.