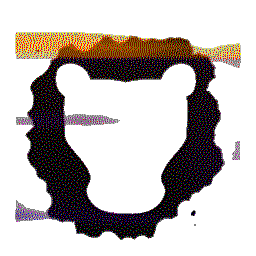 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
28 char pin_key_end,
bool nowait)
34 , pin_key_end_(pin_key_end)
45 using namespace std::chrono;
58 DEBUG(
"This is likely a PIN code");
73 DEBUG(
"DOING SOME CARD READING");
89 using namespace std::chrono;
100 duration_cast<milliseconds>(system_clock::now() -
last_pin_read_);
104 if (elapsed_ms_pin >
delay_)
107 DEBUG(
"PIN READING TIMEOUT");
141 if (with_card && with_pin)
180 assert(bits == 4 || bits == 8);
183 return std::unique_ptr<PinReading>(
188 return std::unique_ptr<PinReading>(
An implementation class that represents a Wiegand Reader.
Autodetect(WiegandReaderImpl *reader, std::chrono::milliseconds delay, char pin_key_end, bool nowait)
Create a strategy that read whatever it can and tries its best to determine what is was that it read.
@ WIEGAND_PIN
Message formatting when using a simple PIN code.
virtual void signal(zmqpp::socket &sock) override
Tells the strategy implementation to send a message to the application containing the received creden...
@ WIEGAND_CARD_PIN
When reading both a card an a PIN code.
TimePoint time_card_read_
virtual void set_reader(WiegandReaderImpl *new_ptr)
Update the pointer that points back to the associated reader.
void read_reset()
Reset the "read state" of the reader, effectively cleaning the wiegand-bit-buffer and resetting the c...
virtual void set_reader(WiegandReaderImpl *new_ptr) override
Update the pointer that points back to the associated reader.
int counter() const
Returns the number of bits read.
PinReadingUPtr read_pin_strategy_
std::chrono::milliseconds delay_
PinReadingUPtr build_strategy(int bits)
Dynamically instanciate a new strategy based on the number of bits available.
The multiple modes available to wiegand reader are implemented through the strategy pattern.
virtual void timeout() override
This is called when the module detect a timeout.
std::unique_ptr< PinReading > PinReadingUPtr
virtual bool completed() const override
Did the strategy gather needed data? If this function returns true, that means that the strategy impl...
CardReadingUPtr read_card_strategy_
Implementation of a wiegand card only strategy.
Provide support for Wiegand devices.
void reset() override
Reset self.
void check_timeout()
Called when timeout() was called but nothing was read.
WiegandReaderImpl * reader_
Strategy for ready PIN only.
const std::string & name() const
Returns the name of this reader.
@ SIMPLE_WIEGAND
This define message formatting for data source SIMPLE_WIEGAND.