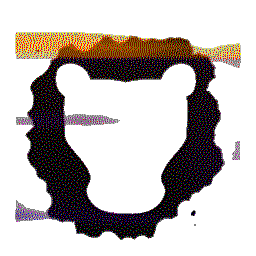 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
44 std::stringstream card_hex;
46 for (std::size_t i = 0; i < size; ++i)
48 card_hex << std::hex << std::setfill(
'0') << std::setw(2)
An implementation class that represents a Wiegand Reader.
virtual void signal(zmqpp::socket &sock) override
Tells the strategy implementation to send a message to the application containing the received creden...
virtual void timeout() override
This is called when the module detect a timeout.
virtual int get_nb_bits() const override
Returns the number of bits in the card.
virtual void reset() override
Reset the strategy, meaning that the next time timeout() is called the behavior should be the same th...
void read_reset()
Reset the "read state" of the reader, effectively cleaning the wiegand-bit-buffer and resetting the c...
int counter() const
Returns the number of bits read.
virtual bool completed() const override
Did the strategy gather needed data? If this function returns true, that means that the strategy impl...
const unsigned char * buffer() const
Return a pointer to internal buffer memory.
Interface for a strategy that read a card number.
virtual const std::string & get_card_id() const override
Returns the card id that was read.
SimpleWiegandStrategy(WiegandReaderImpl *reader)
Provide support for Wiegand devices.
WiegandReaderImpl * reader_
const std::string & name() const
Returns the name of this reader.
@ SIMPLE_WIEGAND
This define message formatting for data source SIMPLE_WIEGAND.