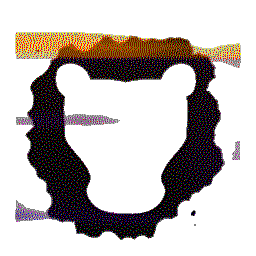 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include "zmqpp/zmqpp.hpp"
24 #include <boost/property_tree/ptree.hpp>
41 class WiegandReaderConfig;
51 const boost::property_tree::ptree &cfg,
CoreUtilsPtr utils);
59 virtual void run()
override;
std::unique_ptr< WiegandConfig > wiegand_config_
Configuration object for the module.
Base class for module implementation.
An implementation class that represents a Wiegand Reader.
std::vector< WiegandReaderImpl > readers_
Vector of wiegand reader managed by this module.
std::unique_ptr< WiegandStrategy > WiegandStrategyUPtr
std::unique_ptr< WSHelperThread > ws_helper_thread_
virtual void run() override
Module's main loop.
This is the header file for a generated source file, GitSHA1.cpp.
~WiegandReaderModule() override
Strategy::WiegandStrategyUPtr create_strategy(const WiegandReaderConfig &reader_config, WiegandReaderImpl *reader)
Internal factory that build a strategy object based upon a reader configuration.
void load_xml_config(const boost::property_tree::ptree &module_config)
Load the module configuration from the XML configuration object.
This simply is the main class for the Wiegand module.
WiegandReaderModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
void process_config()
Create wiegand reader instances based on configuration.
An instance of this class represents the configuration of one Wiegand reader.
std::shared_ptr< CoreUtils > CoreUtilsPtr
void load_db_config()
Load the module configuration from the database.