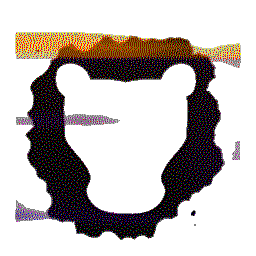 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #define ODB_NO_BASE_VERSION
32 #pragma db model version(1, 1, open)
45 #pragma db object callback(validation_callback) table("HARDWARE_RFIDReader_Wiegand")
49 :
mode(
"SIMPLE_WIEGAND")
59 template <
typename DevicePtrT>
109 "WIEGAND_PIN_BUFFERED",
110 "WIEGAND_CARD_PIN_4BITS",
111 "WIEGAND_CARD_PIN_8BITS",
112 "WIEGAND_CARD_PIN_BUFFERED",
129 const std::vector<WiegandReaderConfigPtr> &
readers()
const;
Hardware::GPIOPtr gpio_low_
std::string buzzer_name() const
std::shared_ptr< GPIO > GPIOPtr
Hardware::BuzzerPtr buzzer_
std::shared_ptr< LED > LEDPtr
Hardware::LEDPtr green_led_
void add_reader(WiegandReaderConfigPtr)
std::string gpio_high_name() const
Transient configuration object that stores the list of reader use/configure.
std::vector< WiegandReaderConfigPtr > readers_
This is the header file for a generated source file, GitSHA1.cpp.
const std::vector< WiegandReaderConfigPtr > & readers() const
std::chrono::milliseconds pin_timeout
void validation_callback(odb::callback_event, odb::database &) const
ODB callback wrt database operation.
constexpr static std::array< const char *const, 8 > valid_operation_modes
List of valid operation mode for a reader.
std::string gpio_low_name() const
Hardware::GPIOPtr gpio_high_
Abstraction of a RFID Reader device.
std::shared_ptr< WiegandReaderConfig > WiegandReaderConfigPtr
An instance of this class represents the configuration of one Wiegand reader.
std::string device_name(const DevicePtrT &dev) const
Returns the name of a device, or the empty string.
std::shared_ptr< Buzzer > BuzzerPtr
std::string green_led_name() const