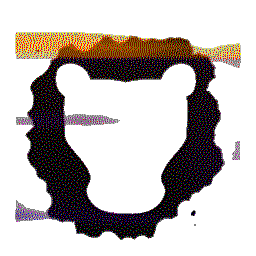 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include "core/audit/DoorEvent_odb.h"
33 , access_point_id_before_(0)
34 , access_point_id_after_(0)
43 ASSERT_LOG(target_door,
"Target door must be non null.");
44 ASSERT_LOG(target_door->id(),
"Target door must be already persisted.");
53 audit->target(target_door);
68 auto door_odb = std::dynamic_pointer_cast<Auth::Door>(door);
69 ASSERT_LOG(door_odb,
"IDoor is not of type Door.");
106 std::stringstream ss;
125 desc[
"alias"] = t->alias();
132 return std::shared_ptr<DoorEvent>(
new DoorEvent());
Auth::DoorId target_door_id_
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
void commit()
Commit the transaction, if there was no currently active transaction at the time of this object's cre...
Provides an implementation of IDoorEvent.
std::shared_ptr< AuditEntry > AuditEntryPtr
static std::shared_ptr< DoorEvent > create_empty()
std::string before_
Optional JSON dump of the object before the event took place.
#define ASSERT_LOG(cond, msg)
void database(DBPtr db)
Set the database pointer.
virtual Auth::AccessPointId access_point_id_before() const override
Return the id of the associated access_point before the event took place.
std::shared_ptr< odb::database > DBPtr
virtual const std::string & after() const override
virtual Auth::DoorId target_id() const override
Auth::AccessPointId access_point_id_after_
The id of associated AP after the event.
std::string generate_target_description() const
Generate a short description for the targeted door.
std::string after_
Optional JSON dump of the object after the event took place.
virtual void target(Auth::IDoorPtr door) override
Set the door that is targeted by the event.
The Audit namespace provides classes and facilities to keep track of what's happening on the Leosac d...
This is the header file for a generated source file, GitSHA1.cpp.
virtual bool finalized() const override
Is this entry finalized.
Auth::AccessPointId access_point_id_before_
The id of the associated AP before the event.
static std::shared_ptr< DoorEvent > create(const DBPtr &database, Auth::IDoorPtr target_door, AuditEntryPtr parent)
std::shared_ptr< IDoor > IDoorPtr
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
virtual Auth::AccessPointId access_point_id_after() const override
Return the id of the associated access_point after the event took place.
virtual AuditEntryId id() const override
Retrieve the identifier of the entry.
virtual const std::string & before() const override
virtual std::string generate_description() const override
Generate a description for this event.
std::shared_ptr< DoorEvent > DoorEventPtr
unsigned long AccessPointId