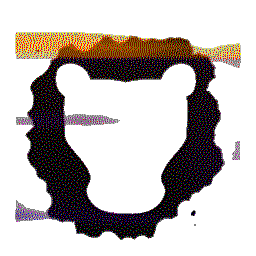 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
21 #include "core/audit/AuditEntry_odb.h"
23 #include "core/auth/User_odb.h"
26 #include <odb/query.hxx>
36 timestamp_ = boost::posix_time::second_clock::local_time();
46 if (e == odb::callback_event::post_update ||
47 e == odb::callback_event::post_persist)
60 "Not currently in a database transaction.");
63 INFO(
"Trying to finalizing already finalized entry. Doing nothing instead.");
91 ASSERT_LOG(user->id(),
"Author is not already persisted.");
97 ASSERT_LOG(
id_,
"Current audit entry must be already persisted.");
101 auto parent_odb = std::dynamic_pointer_cast<AuditEntry>(
parent);
102 ASSERT_LOG(parent_odb,
"Parent is not of type AuditEntry");
104 parent_odb->children_.push_back(shared_from_this());
117 auto &children =
parent->children_;
118 children.erase(std::remove(children.begin(), children.end(), shared_from_this()),
135 ASSERT_LOG(odb::transaction::has_current(),
"Not currently in transaction.");
164 using Query = odb::query<AuditEntry>;
165 Query q(
"ORDER BY id DESC LIMIT 1");
virtual void author(Auth::UserPtr user) override
Set the author of the entry.
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
virtual size_t children_count() const override
Returns the number of children that this entry has.
std::shared_ptr< AuditEntry > AuditEntryPtr
void odb_callback(odb::callback_event e, odb::database &) const
Implementation of an ODB callback.
#define ASSERT_LOG(cond, msg)
virtual Auth::UserId author_id() const override
Retrieve the user id of the author of this entry.
void database(DBPtr db)
Set the database pointer.
std::shared_ptr< User > UserPtr
std::vector< AuditEntryPtr > children_
unsigned long AuditEntryId
std::shared_ptr< odb::database > DBPtr
virtual const EventMask & event_mask() const override
Retrieve the current event mask.
std::shared_ptr< IAuditEntry > IAuditEntryPtr
odb::query< Tools::LogEntry > Query
virtual void remove_parent() override
Remove the parent-child relationship between this entry and its parent.
boost::posix_time::ptime timestamp_
The Audit namespace provides classes and facilities to keep track of what's happening on the Leosac d...
This is the header file for a generated source file, GitSHA1.cpp.
size_t duration_
How long did it take for the Audit object to be finalized.
virtual bool finalized() const override
Is this entry finalized.
virtual void finalize() override
Mark the entry as finalized, and update it wrt the database.
static AuditEntryPtr get_last_audit(DBPtr db)
Retrieve the last (finalized) audit entry.
Tools::ElapsedTimeCounter etc_
Keep track of how long the object has been alive.
FlagSet< EventType > EventMask
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
virtual void set_parent(IAuditEntryPtr parent) override
Set parent as the parent audit entry for this entry.
virtual boost::posix_time::ptime timestamp() const override
Retrieve unix timestamp.
DBPtr database_
Pointer to the database.
bool finalized_
Audit entry are sometime persisted multiple time before reaching their final state.
virtual AuditEntryId id() const override
Retrieve the identifier of the entry.
Implementation of IAuditEntry, backed by ODB.
virtual void reload() override
Reload the object from the database.
virtual size_t version() const override
Returns the ODB version of the object.
Auth::UserLPtr author_
The user at the source of the entry.