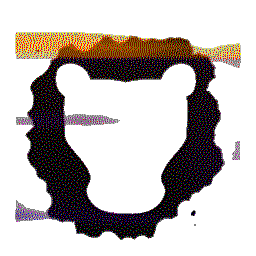 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 #pragma db object polymorphic callback(odb_callback)
40 static std::shared_ptr<DoorEvent>
52 virtual void before(
const std::string &repr)
override;
54 virtual void after(
const std::string &repr)
override;
56 virtual const std::string &
before()
const override;
58 virtual const std::string &
after()
const override;
76 #pragma db on_delete(set_null)
Auth::DoorId target_door_id_
virtual ~DoorEvent()=default
Provides an implementation of IDoorEvent.
std::shared_ptr< AuditEntry > AuditEntryPtr
Interface that describes an Audit object for door related event.
static std::shared_ptr< DoorEvent > create_empty()
std::string before_
Optional JSON dump of the object before the event took place.
void database(DBPtr db)
Set the database pointer.
virtual Auth::AccessPointId access_point_id_before() const override
Return the id of the associated access_point before the event took place.
std::shared_ptr< odb::database > DBPtr
virtual const std::string & after() const override
virtual Auth::DoorId target_id() const override
Auth::AccessPointId access_point_id_after_
The id of associated AP after the event.
std::string generate_target_description() const
Generate a short description for the targeted door.
std::string after_
Optional JSON dump of the object after the event took place.
virtual void target(Auth::IDoorPtr door) override
Set the door that is targeted by the event.
This is the header file for a generated source file, GitSHA1.cpp.
Auth::AccessPointId access_point_id_before_
The id of the associated AP before the event.
Provide static methods to instanciate various Audit objects.
static std::shared_ptr< DoorEvent > create(const DBPtr &database, Auth::IDoorPtr target_door, AuditEntryPtr parent)
std::shared_ptr< IDoor > IDoorPtr
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
virtual Auth::AccessPointId access_point_id_after() const override
Return the id of the associated access_point after the event took place.
Implementation of IAuditEntry, backed by ODB.
virtual const std::string & before() const override
virtual std::string generate_description() const override
Generate a description for this event.
unsigned long AccessPointId
odb::lazy_weak_ptr< Door > DoorLWPtr