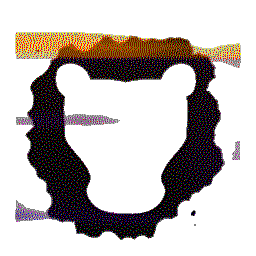 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
20 #include "core/auth/Zone_odb.h"
30 , type_(Type::LOGICAL)
102 if (e == odb::callback_event::post_update ||
103 e == odb::callback_event::post_persist)
105 std::set<ZoneId> ids;
110 static void insert_enforce_unique(std::set<ZoneId> &zone_ids,
ZoneId id)
112 auto inserted = zone_ids.insert(
id);
113 if (!inserted.second)
117 "There cannot be cycle in parent/child relationship.");
123 unsigned int physical_parent_count = 0;
127 insert_enforce_unique(zone_ids, z.
id());
130 for (
auto &lazy_parent : z.
parents_)
132 auto parent(lazy_parent.load());
136 ++physical_parent_count;
144 "",
"A physical zone cannot have more than one physical parent.");
150 "A logical zone cannot have physical zone as a parent");
156 auto child(lazy_children.load());
171 throw ModelException(
"data/attributes/type",
"Invalid zone type.");
virtual Type type() const override
virtual void add_door(DoorLPtr door) override
virtual std::vector< ZoneLPtr > children() const override
Retrieve the children zones.
virtual std::string alias() const override
#define ASSERT_LOG(cond, msg)
virtual void clear_doors() override
virtual std::vector< DoorLPtr > doors() const override
Retrieve the doors associated with the zones.
std::vector< ZoneLPtr > children_
std::vector< ZoneLWPtr > parents_
virtual std::string description() const override
A Zone is a container for doors and other zone.
This is the header file for a generated source file, GitSHA1.cpp.
static void validate_type(IZone::Type value)
Validate that the integer value of the type is a correct type for a zone.
virtual void clear_children() override
virtual ZoneId id() const override
virtual void add_child(ZoneLPtr zone) override
void validation_callback(odb::callback_event e, odb::database &) const
Callback function called by ODB before/after database operation against a Zone object.
An exception class for general API error.
odb::lazy_shared_ptr< Zone > ZoneLPtr
static void validate(const Zone &z, std::set< ZoneId > &zone_ids)
Perform validation of the zone.
odb::lazy_shared_ptr< Door > DoorLPtr
std::vector< DoorLPtr > doors_