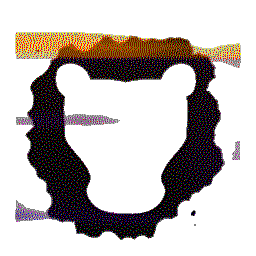 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
47 class IZone :
public std::enable_shared_from_this<IZone>
60 virtual std::string
alias()
const = 0;
64 virtual void alias(
const std::string &
alias) = 0;
65 virtual void description(
const std::string &desc) = 0;
77 virtual std::vector<ZoneLPtr>
children()
const = 0;
82 virtual std::vector<DoorLPtr>
doors()
const = 0;
virtual void clear_children()=0
virtual std::vector< ZoneLPtr > children() const =0
Retrieve the children zones.
virtual std::string description() const =0
virtual std::vector< DoorLPtr > doors() const =0
Retrieve the doors associated with the zones.
This is the header file for a generated source file, GitSHA1.cpp.
virtual std::string alias() const =0
virtual void clear_doors()=0
odb::lazy_shared_ptr< Zone > ZoneLPtr
virtual Type type() const =0
virtual void add_child(ZoneLPtr zone)=0
virtual ZoneId id() const =0
odb::lazy_shared_ptr< Door > DoorLPtr
virtual void add_door(DoorLPtr door)=0