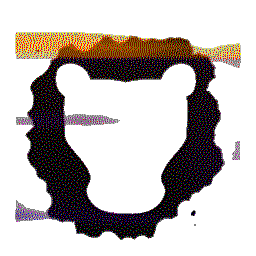 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include "core/auth/Door_odb.h"
23 #include "core/auth/Zone_odb.h"
32 json serialized = {{
"id", zone.
id()},
35 {{
"alias", zone.
alias()},
37 {
"type",
static_cast<int>(zone.
type())}}}};
39 json children = json::array();
42 json child_zone_info = {{
"id", lazy_zone.object_id()}, {
"type",
"zone"}};
43 children.push_back(child_zone_info);
46 json doors = json::array();
49 json door_info = {{
"id", lazy_door.object_id()}, {
"type",
"door"}};
50 doors.push_back(door_info);
53 serialized[
"relationships"] = {{
"children", {{
"data", children}}},
54 {
"doors", {{
"data", doors}}}};
68 auto doors_ids = in.at(
"doors");
70 for (
const auto &door_id : doors_ids)
76 auto children_ids = in.at(
"children");
78 for (
const auto &child_id : children_ids)
94 auto tmp = json::parse(in);
Holds classes relevant to the Authentication and Authorization subsystem.
Add a few useful extraction functions.
std::chrono::system_clock::time_point extract_with_default(const nlohmann::json &obj, const std::string &key, const std::chrono::system_clock::time_point &tp)
Extract an ISO 8601 datetime string from a json object.
virtual void clear_children()=0
static json serialize(const Auth::IZone &Zone, const SecurityContext &sc)
virtual std::vector< ZoneLPtr > children() const =0
Retrieve the children zones.
virtual std::string description() const =0
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
virtual std::vector< DoorLPtr > doors() const =0
Retrieve the doors associated with the zones.
Provides various database-related services to consumer.
This is the header file for a generated source file, GitSHA1.cpp.
static std::string serialize(const Auth::IZone &in, const SecurityContext &sc)
static void unserialize(Auth::IZone &out, const std::string &in, const SecurityContext &sc)
virtual std::string alias() const =0
virtual void clear_doors()=0
static void unserialize(Auth::IZone &out, const json &in, const SecurityContext &sc)
odb::lazy_shared_ptr< Zone > ZoneLPtr
std::shared_ptr< ServiceInterface > get_service() const
Retrieve the service instance implementing the ServiceInterface, or nullptr if no such service was re...
virtual Type type() const =0
virtual void add_child(ZoneLPtr zone)=0
virtual ZoneId id() const =0
odb::lazy_shared_ptr< Door > DoorLPtr
A SecurityContext is used to query permission while doing an operation.
virtual void add_door(DoorLPtr door)=0