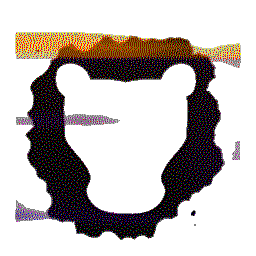 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #include "core/auth/Group_odb.h"
27 #include "core/auth/Token_odb.h"
29 #include "core/auth/UserGroupMembership_odb.h"
30 #include "core/auth/User_odb.h"
36 #include <odb/session.hxx>
61 std::string username = req.at(
"username");
62 std::string password = req.at(
"password");
69 rep[
"user_id"] = token->owner()->id();
70 rep[
"token"] = token->token();
76 rep[
"message"] =
"Invalid credentials";
91 rep[
"user_id"] = token->owner()->id();
92 rep[
"username"] = token->owner()->username();
98 rep[
"message"] =
"Invalid credentials";
118 rep[
"instance_name"] = core_api.instance_name();
119 rep[
"config_version"] = core_api.config_version();
120 rep[
"uptime"] = core_api.uptime();
121 rep[
"modules"] = core_api.modules_names();
128 if (cmd ==
"get_leosac_version")
130 if (cmd ==
"create_auth_token" || cmd ==
"authenticate_with_token")
139 odb::core::transaction t(
server_.
db()->begin());
146 catch (
const odb::object_changed &e)
195 std::make_unique<UserSecurityContext>(
server_.
dbsrv(), token->owner()->id());
void clear_authentication()
A SecurityContext with no permission.
void hook_before_request()
A hook that is called before a request processing method will be invoked.
json get_leosac_version(const json &)
Retrieve the current version number of Leosac.
Auth::UserPtr current_user() const
Retrieve the user associated with the session, or nullptr.
std::shared_ptr< Token > TokenPtr
Auth::TokenPtr authenticate_token(const std::string &token_str) const
Attempt to authenticate with an authentication token.
#define ASSERT_LOG(cond, msg)
std::shared_ptr< User > UserPtr
APIAuth & auth()
Retrieve the authentication helper.
Acts like an odb::session, with the exception that it will save the current active session (if any) a...
APISession(WSServer &server)
std::unique_ptr< SecurityContext > security_
bool allowed(const std::string &cmd)
Is this API client allowed to perform the request cmd ?
void mark_authenticated(Auth::TokenPtr token)
Auth::UserId current_user_id() const
Retrieve the UserId of the user associated with this API session.
Auth::TokenPtr authenticate_credentials(const std::string &username, const std::string &password) const
Attempt to authenticate with username/password credential and generate an authentication token.
All modules that provides features to Leosac shall be in this namespace.
json logout(const json &req)
Log an user out.
json system_overview(const json &req)
Presents an overview of the system to the end user.
This is the header file for a generated source file, GitSHA1.cpp.
WSServer & server_
The API server.
The implementation class that runs the websocket server.
AuthStatus
Enumeration describing the authentication status of a client.
void invalidate_token(Auth::TokenPtr token) const
Invalidate the authentication token, removing it from the database.
CoreUtilsPtr core_utils()
Retrieve the CoreUtils pointer.
Auth::TokenPtr current_token() const
Retrieve the currently in-use token, or nullptr.
DBPtr db()
Retrieve database handle.
SecurityContext & security_context() const
json authenticate_with_token(const json &req)
Attempt to authenticate with a (previously generated) authentication token.
DBServicePtr dbsrv()
Retrieve database service pointer.
json create_auth_token(const json &req)
Generate an authentication token using the user credential, and logs the user in on success.
Auth::TokenPtr current_auth_token_
The token we are authenticated with.
void abort_session()
Abort the current websocket session.
A SecurityContext is used to query permission while doing an operation.