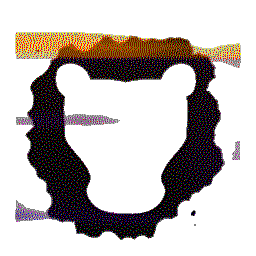 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include <boost/algorithm/string.hpp>
46 auto card_num_hex = boost::replace_all_copy(
card_id_,
":",
"");
48 static_assert(
sizeof(decltype(std::stoull(card_num_hex,
nullptr, 16))) ==
50 "stoul not big enough");
52 uint64_t tmp = std::stoull(card_num_hex,
nullptr, 16);
53 int trailing_zero = (64 -
nb_bits_) % 8;
54 tmp >>= trailing_zero;
67 INFO(
"Not using format to convert WiegandCard to integer because no format "
76 assert(
card_id_.size() == 2 * 4 + 3);
89 assert(
card_id_.size() == 2 * 5 + 4);
121 std::istringstream ss(card_id);
125 for (
int i = 0; i < 2; ++i)
128 if (!isxdigit(c) || !ss.good())
140 "Card id must have aa:bb:cc:11 format.");
149 "The number of bits must be > 0");
virtual CredentialId id() const override
Retrieve the identifier of the credential.
static void validate(const IRFIDCard &card)
uint64_t to_wiegand_34() const
Extract the card ID, assuming the format to be Wiegand34.
virtual int nb_bits() const override
virtual const std::string & card_id() const =0
static void validate_nb_bits(int nb_bits)
This is the header file for a generated source file, GitSHA1.cpp.
virtual int nb_bits() const =0
static void validate_card_id(const std::string &card_id)
virtual const std::string & card_id() const override
uint64_t to_wiegand_26() const
Extract the card ID, assuming the format to be Wiegand26.
An exception class for general API error.
virtual uint64_t to_int() const override
Returns the integer representation of the card ID.
virtual uint64_t to_raw_int() const override
Convert the bits of the card to an integer.
Interface for RFIDCard credential.