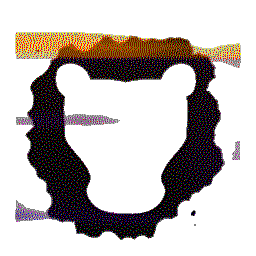 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 #pragma db object polymorphic optimistic
48 virtual const std::string &
card_id()
const override;
50 virtual int nb_bits()
const override;
52 virtual uint64_t
to_int()
const override;
58 void card_id(
const std::string &
string)
override;
MAKE_VISITABLE_FALLBACK(IRFIDCard)
static void validate(const IRFIDCard &card)
uint64_t to_wiegand_34() const
Extract the card ID, assuming the format to be Wiegand34.
virtual int nb_bits() const override
static void validate_nb_bits(int nb_bits)
This is the header file for a generated source file, GitSHA1.cpp.
static void validate_card_id(const std::string &card_id)
An ODB enabled credential object.
virtual const std::string & card_id() const override
uint64_t to_wiegand_26() const
Extract the card ID, assuming the format to be Wiegand26.
virtual uint64_t to_int() const override
Returns the integer representation of the card ID.
virtual uint64_t to_raw_int() const override
Convert the bits of the card to an integer.
Interface for RFIDCard credential.