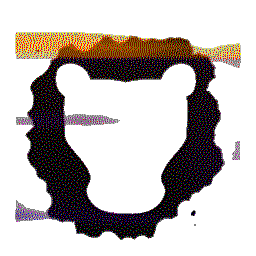 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
59 bool abort_on_failure)
61 using VisitedT = std::remove_reference_t<std::remove_const_t<T>>;
85 #define MAKE_VISITABLE() \
86 virtual void accept(::Leosac::Tools::BaseVisitor &v) override \
88 visitor_dispatch(*this, v, true); \
90 virtual void accept(::Leosac::Tools::BaseVisitor &v) const override \
92 visitor_dispatch(*this, v, true); \
104 #define MAKE_VISITABLE_FALLBACK(PARENT_CLASS) \
105 virtual void accept(::Leosac::Tools::BaseVisitor &v) override \
107 if (!visitor_dispatch(*this, v, false)) \
109 PARENT_CLASS::accept(v); \
112 virtual void accept(::Leosac::Tools::BaseVisitor &v) const override \
114 if (!visitor_dispatch(*this, v, false)) \
116 PARENT_CLASS::accept(v); \
This is the header file for a generated source file, GitSHA1.cpp.