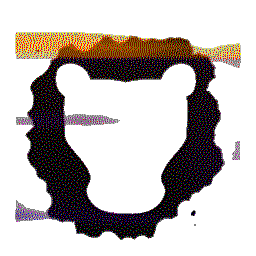 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
21 #include "core/audit/GroupEvent_odb.h"
35 ASSERT_LOG(target_group,
"Target group must be non null.");
36 ASSERT_LOG(target_group->id(),
"Target group must be already persisted.");
45 audit->target(target_group);
97 using namespace FlagSetOperator;
117 desc[
"name"] = t->name();
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
void commit()
Commit the transaction, if there was no currently active transaction at the time of this object's cre...
std::shared_ptr< AuditEntry > AuditEntryPtr
#define ASSERT_LOG(cond, msg)
void database(DBPtr db)
Set the database pointer.
std::shared_ptr< odb::database > DBPtr
std::string generate_description() const override
Generate a description for this event.
std::shared_ptr< GroupEvent > GroupEventPtr
const std::string & before() const override
The Audit namespace provides classes and facilities to keep track of what's happening on the Leosac d...
This is the header file for a generated source file, GitSHA1.cpp.
static std::shared_ptr< GroupEvent > create(const DBPtr &database, Auth::GroupPtr target_group, AuditEntryPtr parent)
virtual bool finalized() const override
Is this entry finalized.
std::shared_ptr< Group > GroupPtr
Auth::GroupId target_id() const override
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
Provides an implementation of IGroupEvent.
Auth::GroupId target_group_id_
This is equals to target_->id().
static std::shared_ptr< GroupEvent > create_empty()
std::string generate_target_description() const
Generate a small json-string description about the targeted group.
virtual void target(Auth::GroupPtr grp) override
Set the group that is targeted by the event.
std::string after_
Optional JSON dump of the object after the event took place.
const std::string & after() const override
std::string before_
Optional JSON dump of the object before the event took place.