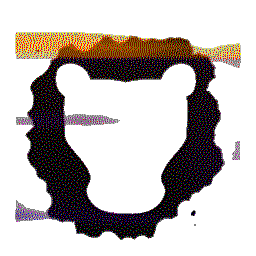 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 #pragma db object polymorphic callback(odb_callback)
40 static std::shared_ptr<GroupEvent>
50 virtual void before(
const std::string &repr)
override;
52 virtual void after(
const std::string &repr)
override;
56 const std::string &
before()
const override;
58 const std::string &
after()
const override;
69 #pragma db on_delete(set_null)
virtual ~GroupEvent()=default
std::shared_ptr< AuditEntry > AuditEntryPtr
Interface that describes an Audit object for group related event.
void database(DBPtr db)
Set the database pointer.
std::shared_ptr< odb::database > DBPtr
std::string generate_description() const override
Generate a description for this event.
const std::string & before() const override
This is the header file for a generated source file, GitSHA1.cpp.
static std::shared_ptr< GroupEvent > create(const DBPtr &database, Auth::GroupPtr target_group, AuditEntryPtr parent)
Provide static methods to instanciate various Audit objects.
std::shared_ptr< Group > GroupPtr
Auth::GroupId target_id() const override
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
Provides an implementation of IGroupEvent.
Auth::GroupId target_group_id_
This is equals to target_->id().
static std::shared_ptr< GroupEvent > create_empty()
std::string generate_target_description() const
Generate a small json-string description about the targeted group.
virtual void target(Auth::GroupPtr grp) override
Set the group that is targeted by the event.
Implementation of IAuditEntry, backed by ODB.
std::string after_
Optional JSON dump of the object after the event took place.
odb::lazy_weak_ptr< Group > GroupLWPtr
const std::string & after() const override
std::string before_
Optional JSON dump of the object before the event took place.