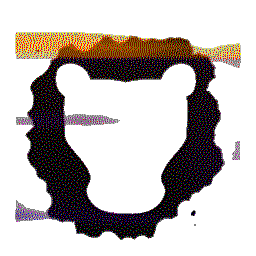 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
33 std::thread(std::bind(&
Task::run, t)).detach();
37 std::lock_guard<std::mutex> lg(mutex_);
38 queues_[policy].push(t);
45 auto &queue = queues_[me];
46 int run = queue.size();
52 auto task = queue.front();
62 std::lock_guard<std::mutex> lg(mutex_);
63 assert(queues_.count(me) == 0);
Scheduler(Kernel *kptr)
Construct a scheduler object (generally 1 per application).
void update(TargetThread me) noexcept
This will run queued tasks that are scheduled to run on thread me.
This is the header file for a generated source file, GitSHA1.cpp.
void register_thread(TargetThread me)
This is currently useless.
std::shared_ptr< Task > TaskPtr
Kernel & kernel()
Retrieve the kernel reference associated with the scheduler.
std::enable_if< !std::is_convertible< Callable, std::shared_ptr< Tasks::Task > >::value, void >::type enqueue(const Callable &call, TargetThread policy)