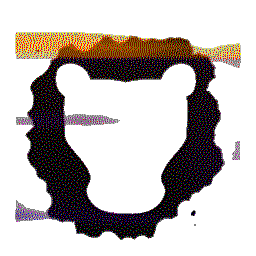 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
63 template <
typename Callable>
64 typename std::enable_if<
65 !std::is_convertible<Callable, std::shared_ptr<Tasks::Task>>::value,
Scheduler(Kernel *kptr)
Construct a scheduler object (generally 1 per application).
std::map< TargetThread, TaskQueue > TaskQueueMap
std::queue< Tasks::TaskPtr > TaskQueue
Scheduler & operator=(const Scheduler &)=delete
void update(TargetThread me) noexcept
This will run queued tasks that are scheduled to run on thread me.
TaskQueueMap queues_
The internal queues of tasks.
This is the header file for a generated source file, GitSHA1.cpp.
void register_thread(TargetThread me)
This is currently useless.
This is a scheduler that is used internally to schedule asynchronous / long running tasks.
static TaskPtr build(const Callable &callable)
std::shared_ptr< Task > TaskPtr
Kernel & kernel()
Retrieve the kernel reference associated with the scheduler.
std::enable_if< !std::is_convertible< Callable, std::shared_ptr< Tasks::Task > >::value, void >::type enqueue(const Callable &call, TargetThread policy)