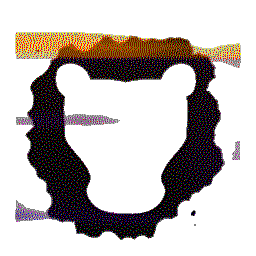 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
20 #include "core/auth/Group_odb.h"
21 #include "core/auth/UserGroupMembership_odb.h"
50 auto ptr = membership->user().get_eager().lock();
65 auto ugm = std::make_shared<UserGroupMembership>();
68 ugm->group(shared_from_this());
99 "Membership doesn't point to self.");
100 ASSERT_LOG(membership->user().lock(),
"Why is this null?");
101 if (membership->user().lock())
102 members.push_back(membership->user().lock());
115 if (e == odb::callback_event::post_update ||
116 e == odb::callback_event::post_persist)
120 if (membership->id() == 0)
121 db.persist(membership);
123 db.update(membership);
147 if (membership->user().object_id() == user_id)
150 *rank_out = membership->rank();
174 if (name.size() < 3 || name.size() > 50)
176 throw ModelException(
"data/attributes/name",
"Length must be >=3 and <=50.");
178 for (
const auto &c : name)
180 if (!isalnum(c) && (c !=
'_' && c !=
'-' && c !=
'.'))
183 "data/attributes/name",
184 BUILD_STR(
"Usage of unauthorized character: " << c));
const UserGroupMembershipSet & user_memberships() const
Retrieve the UserGroupMembership that this group is involved with.
Holds classes relevant to the Authentication and Authorization subsystem.
#define BUILD_STR(param)
Internal macro.
IAccessProfilePtr profile()
GroupId id() const
Retrieve the unique identifier of the group.
#define ASSERT_LOG(cond, msg)
UserGroupMembershipSet membership_
std::vector< Tools::ScheduleMappingLWPtr > schedules_mapping_
std::shared_ptr< User > UserPtr
std::string description_
A (potentially long) description of the group.
std::shared_ptr< IAccessProfile > IAccessProfilePtr
std::vector< Tools::ScheduleMappingLWPtr > lazy_schedules_mapping() const
Returns the vector of lazy_weak_ptr to schedule mapping.
void odb_callback(odb::callback_event e, odb::database &) const
GroupId id_
The group identifier.
This is the header file for a generated source file, GitSHA1.cpp.
static void validate_name(const std::string &name)
UserGroupMembershipPtr member_add(UserPtr m, GroupRank rank=GroupRank::MEMBER)
A authentication group regroup users that share permissions.
bool member_has(Auth::UserId user_id, GroupRank *rank_out=nullptr) const
Check if user_id is a member of this group.
const std::string & name() const
std::shared_ptr< UserGroupMembership > UserGroupMembershipPtr
IAccessProfilePtr profile_
An exception class for general API error.
std::vector< UserPtr > loaded_members_
This returns a vector of loaded User object.
std::set< UserGroupMembershipPtr, UserGroupMembershipComparator > UserGroupMembershipSet
std::vector< UserLPtr > lazy_members() const
Retrieve lazy pointers to members.
GroupRank
The rank of an User inside a Group.
void schedule_mapping_added(const Tools::ScheduleMappingPtr &sched_mapping)
The group has been mapped by a schedule.
const std::vector< UserPtr > & members() const
static void validate(const Group &grp)
Validate the group's attributes.
const std::string & description() const