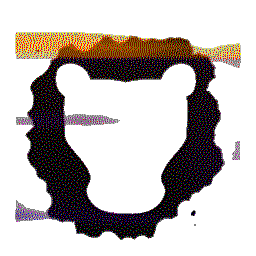 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
29 , bus_sub_(ctx,
zmqpp::socket_type::sub)
30 , contact_triggered(false)
32 bus_sub_.connect(
"inproc://zmq-bus-pub");
34 if (
door->exitreq_gpio())
39 if (
door->contact_gpio())
std::chrono::system_clock::time_point contact_lastupdate
A Facade to a GPIO object.
std::shared_ptr< AuthTarget > AuthTargetPtr
std::string topic_exitreq_
DoormanDoor(const Auth::AuthTargetPtr &door, zmqpp::context &ctx)
Create a new doorman door.
Leosac::Auth::AuthTargetPtr door() const
Leosac::Auth::AuthTargetPtr door_
void handle_bus_msg()
Activity we care about happened on the bus.
zmqpp::socket & bus_sub()
Module that allows user to configure action to be taken to react to messages from other modules.
bool turnOn(std::chrono::milliseconds duration)
Turn the GPIO ON and turn it OFF duration milliseconds later.
std::string topic_contact_