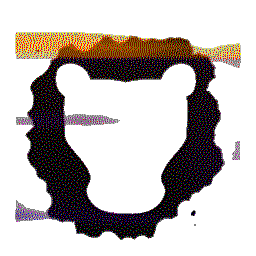 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
27 template <
unsigned int NbBits>
32 , pin_timeout_(pin_timeout)
33 , pin_end_key_(pin_end_key)
39 template <
unsigned int NbBits>
50 DEBUG(
"Buffer value = " << (
unsigned int)input);
52 for (
int i = 0; i < 4; ++i)
54 bool v = ((input >> (7 - i)) & 0x01);
67 return std::to_string(n).at(0);
70 template <
unsigned int NbBits>
73 static_assert(NbBits == 4 || NbBits == 8,
74 "Must either be 4 or 8 bits per key pressed");
75 using namespace std::chrono;
77 duration_cast<milliseconds>(system_clock::now() - last_update_);
79 if (!reader_->counter())
81 if (elapsed_ms > pin_timeout_)
86 if (reader_->counter() != NbBits)
88 WARN(
"Expected number of bits invalid. ("
89 << reader_->counter() <<
" but we expected " << NbBits <<
")");
94 last_update_ = system_clock::now();
95 char c = extract_from_raw(reader_->buffer()[0]);
96 if (c == pin_end_key_)
102 reader_->read_reset();
105 template <
unsigned int NbBits>
108 if (inputs_.length())
114 template <
unsigned int NbBits>
120 template <
unsigned int NbBits>
124 assert(inputs_.length());
125 DEBUG(
"Sending PIN Code: " << inputs_);
133 template <
unsigned int NbBits>
139 template <
unsigned int NbBits>
142 reader_->read_reset();
145 last_update_ = std::chrono::system_clock::now();
char extract_from_raw(uint8_t input) const
Extract the character that was pressed from raw data.
WiegandPinNBitsOnly(WiegandReaderImpl *reader, std::chrono::milliseconds pin_timeout, char pin_end_key)
Create a strategy that read N bits-per-key PIN code.
An implementation class that represents a Wiegand Reader.
@ WIEGAND_PIN
Message formatting when using a simple PIN code.
Interface for a strategy that read a PIN code.
virtual void timeout() override
This is called when the module detect a timeout.
virtual void reset() override
Reset the strategy, meaning that the next time timeout() is called the behavior should be the same th...
This is the header file for a generated source file, GitSHA1.cpp.
virtual const std::string & get_pin() const override
Retrieve the pin code that was read from the reader.
void end_of_input()
Timeout or pin_end_key read.
virtual bool completed() const override
Did the strategy gather needed data? If this function returns true, that means that the strategy impl...
Provide support for Wiegand devices.
Strategy for ready PIN only.
virtual void signal(zmqpp::socket &sock) override
Tells the strategy implementation to send a message to the application containing the received creden...