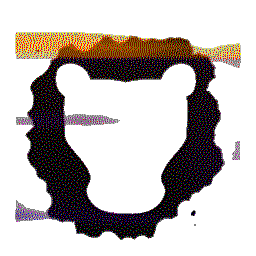 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
29 #include <boost/asio.hpp>
60 template <
typename ParameterT>
65 const ParameterT ¶m)
79 catch (
const std::exception &e)
81 ERROR(
"Failed to join WebSockAPI::BaseModuleSupportThread");
116 io_.post([
this, ws_service]() {
122 work_ = std::make_unique<boost::asio::io_service::work>(
io_);
131 auto &service_registered_event =
132 assert_cast<const service_event::ServiceRegistered &>(e);
133 if (service_registered_event.interface_type() ==
134 boost::typeindex::type_id<WebSockAPI::Service>())
140 ASSERT_LOG(ws_service,
"WSService has disappeared");
163 boost::asio::io_service
io_;
164 std::unique_ptr<boost::asio::io_service::work>
work_;
boost::asio::io_service io_
void set_parameter(const ParameterT &p)
std::unique_ptr< boost::asio::io_service::work > work_
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
void start_running()
Effectively starts an helper thread and run its io_service.
#define ASSERT_LOG(cond, msg)
This class provide a base implementation -designed to be extended by subclass- so that non-boost-asio...
BaseModuleSupportThread(const CoreUtilsPtr &core_utils, const ParameterT ¶m)
std::shared_ptr< WebSockAPI::Service > service_ptr_
This is the header file for a generated source file, GitSHA1.cpp.
A service object provided by the Websocket module.
virtual ~BaseModuleSupportThread()
void on_service_event(const service_event::Event &e)
virtual void register_ws_handlers(Service &ws_service)=0
Called when websocket handler registration is possible.
virtual void unregister_ws_handlers(Service &ws_service)=0
bs2::connection register_event_listener(T &&callable)
Register a service-event listener.
std::shared_ptr< ServiceInterface > get_service() const
Retrieve the service instance implementing the ServiceInterface, or nullptr if no such service was re...
bs2::scoped_connection service_event_listener_
std::unique_ptr< std::thread > thread_
std::shared_ptr< CoreUtils > CoreUtilsPtr