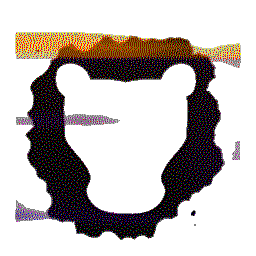 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
29 #include <boost/asio/io_service.hpp>
30 #include <boost/optional.hpp>
61 template <
typename HandlerT>
63 boost::asio::io_service &io)
72 [&, handler](
const RequestContext &req_ctx) -> boost::optional<json> {
73 std::packaged_task<boost::optional<json>(
const RequestContext &)> pt(
75 auto future = pt.get_future();
76 io.post([&]() { pt(req_ctx); });
87 template <
typename HandlerT>
111 template <
typename HandlerT>
113 const std::string &type,
115 boost::asio::io_service &io)
117 auto wrapped = [handler, permission](
const RequestContext &req_ctx) {
118 req_ctx.security_ctx.enforce_permission(permission.first,
120 return handler(req_ctx);
125 template <
typename HandlerT>
127 const std::string &type,
129 boost::asio::io_service &io)
132 aap.first = permission;
bool register_typed_handler(const WSHandler &handler, const std::string &type)
Register an handler that is ready to be invoked in the websocket thread.
bool register_asio_handler_permission(HandlerT &&handler, const std::string &type, SecurityContext::Action permission, boost::asio::io_service &io)
void register_crud_handler(const std::string &resource_name, CRUDResourceHandler::Factory factory)
CRUDResourceHandlerUPtr(*)(RequestContext) Factory
bool register_asio_handler(HandlerT &&handler, const std::string &type, boost::asio::io_service &io)
Register an handler that will be invoked by the io_service io.
std::pair< SecurityContext::Action, SecurityContext::ActionParam > ActionActionParam
A pair of action and their parameters.
This is the header file for a generated source file, GitSHA1.cpp.
A service object provided by the Websocket module.
std::function< boost::optional< json >(const RequestContext &)> WSHandler
The implementation class that runs the websocket server.
bool register_handler(HandlerT &&handler, const std::string &type)
Register a handler for a websocket message.
Service(WSServer &server)
void unregister_handler(const std::string &name)
Remove an handler by name.
Holds valuable pointer to provide context to a request.
bool register_asio_handler_permission(HandlerT &&handler, const std::string &type, ActionActionParam permission, boost::asio::io_service &io)