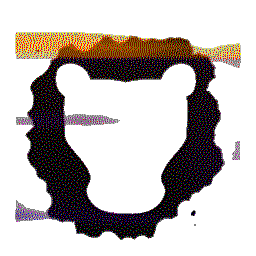 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include <boost/filesystem.hpp>
26 #include <zmqpp/proxy.hpp>
33 const boost::property_tree::ptree &cfg,
37 port_ = cfg.get<uint16_t>(
"module_config.port", 8976);
38 interface_ = cfg.get<std::string>(
"module_config.interface",
"127.0.0.1");
43 << endpoint_colorized);
55 INFO(
"Websocket module registered shutdown. Will now stop websocket server.");
WebSockAPIModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
Base class for module implementation.
virtual void run() override
This is the main loop of the module.
void run(const std::string &interface, uint16_t port)
All modules that provides features to Leosac shall be in this namespace.
std::string underline(const T &in)
CoreUtilsPtr core_utils()
This module explicitly expose CoreUtils to other object in the module.
uint16_t port_
Port to bind the websocket endpoint.
This is the header file for a generated source file, GitSHA1.cpp.
zmqpp::reactor reactor_
The reactor object we poll() on in the main loop.
CoreUtilsPtr utils_
Pointer to the core utils, which gives access to scheduler and others.
std::string green(const T &in)
bool is_running_
Boolean indicating whether the main loop should run or not.
std::string format(const std::string &escape_code, const T &in)
Return a string containing the escape code, a string representation of T and the clear escape string.
std::string interface_
IP address of the interface to listen on.
std::unique_ptr< WSServer > wssrv_
Our websocket server object.
std::shared_ptr< CoreUtils > CoreUtilsPtr