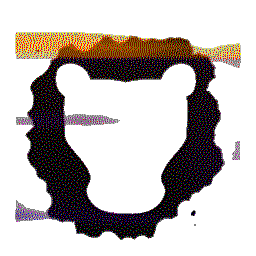 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
41 boost::property_tree::ptree cfg, module_cfg, leds_cfg, led1_cfg;
43 led1_cfg.add(
"name",
"my_led");
44 led1_cfg.add(
"gpio",
"my_gpio");
45 led1_cfg.add(
"default_blink_duration",
"1000");
46 led1_cfg.add(
"default_blink_speed",
"100");
48 leds_cfg.add_child(
"led", led1_cfg);
49 module_cfg.add_child(
"leds", leds_cfg);
51 cfg.add(
"name",
"LED_BUZZER");
52 cfg.add_child(
"module_config", module_cfg);
54 return test_run_module<LEDBuzzerModule>(&ctx_, pipe, cfg);
60 , gpio_(ctx_,
"my_gpio")
61 , gpio_actor_(std::bind(&
FakeGPIO::run, &gpio_, std::placeholders::_1))
63 bus_sub_.subscribe(
"");
79 FLED my_led(ctx_,
"my_led");
80 ASSERT_TRUE(my_led.
isOff());
82 ASSERT_TRUE(my_led.
turnOn());
83 ASSERT_TRUE(my_led.
isOn());
84 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"ON"));
89 FLED my_led(ctx_,
"my_led");
90 ASSERT_TRUE(my_led.
isOff());
92 ASSERT_TRUE(my_led.
turnOn());
93 ASSERT_TRUE(my_led.
isOn());
94 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"ON"));
97 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"OFF"));
98 ASSERT_TRUE(my_led.
isOff());
103 FLED my_led(ctx_,
"my_led");
104 ASSERT_TRUE(my_led.
isOff());
107 ASSERT_TRUE(my_led.
toggle());
108 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"ON"));
109 ASSERT_TRUE(my_led.
isOn());
111 ASSERT_TRUE(my_led.
toggle());
112 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"OFF"));
113 ASSERT_TRUE(my_led.
isOff());
121 FLED my_led(ctx_,
"my_led");
122 ASSERT_TRUE(my_led.
isOff());
123 ASSERT_TRUE(my_led.
state().
st == FLED::State::OFF);
128 ASSERT_TRUE(my_led.
state().
st == FLED::State::BLINKING);
130 for (
int i = 0; i < 5; ++i)
132 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"ON"));
133 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"OFF"));
135 ASSERT_TRUE(my_led.
isOff());
136 ASSERT_EQ(FLED::State::OFF, my_led.
state().
st);
145 FLED my_led(ctx_,
"my_led");
147 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"ON"));
148 ASSERT_TRUE(my_led.
isOn());
149 ASSERT_TRUE(my_led.
state().
st == FLED::State::ON);
154 ASSERT_TRUE(my_led.
state().
st == FLED::State::BLINKING);
156 for (
int i = 0; i < 4; ++i)
158 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"OFF"));
159 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"ON"));
161 ASSERT_TRUE(
bus_read(bus_sub_,
"S_my_gpio",
"OFF"));
162 ASSERT_TRUE(my_led.
isOff());
163 ASSERT_EQ(FLED::State::OFF, my_led.
state().
st);
171 FLED my_led(ctx_,
"my_led");
172 ASSERT_TRUE(my_led.
isOff());
173 ASSERT_TRUE(my_led.
state().
st == FLED::State::OFF);
176 my_led.
blink(100, 10);
178 ASSERT_TRUE(my_led.
state().
st == FLED::State::BLINKING);
int64_t speed
Set only if st is BLINKING, it represents the speed of blinking.
RuntimeOptions class declaration.
enum Leosac::Hardware::FLED::State::@0 st
Internal state of the LED.
bool turnOff()
Turn the LED OFF by sending a message to the backend LED impl.
bool isOff()
Similar to isOn().
A Facade to a LED object.
TEST_F(LedTest, blink3)
Test regression for #61.
bool isOn()
Query the value of the GPIO and returns true if the LED is ON.
This is the header file for a generated source file, GitSHA1.cpp.
State state()
Return the state of the device.
Namespace where implementation of Led (or buzzer) support is done.
bool toggle()
Toggle the LED value by sending a message to the backend LED impl.
bool turnOn(int duration)
Turn the LED ON and turn it OFF duration milliseconds later.
bool bus_read(zmqpp::socket &sub, Content... content)
Make a blocking read on the bus, return true if content match the message.
Unit testing utility class that helps writing test.
bool isBlinking()
Returns true is the LED is currently blinking.
int64_t duration
Set only if st is BLINKING, it represents the total duration of blinking.
virtual bool run_module(zmqpp::socket *pipe) override
Called in the module's actor's thread.
Base class for test fixtures, it defines a ZMQ context, a BUS and a sub socket connect to the bus.
bool blink()
Make the LED blink.
Provides facade classes to hardware device implementation.
A test helper class that emulate a GPIO pin.