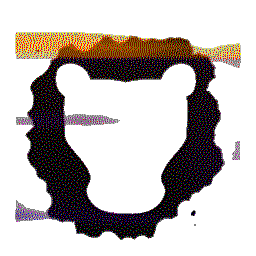 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include <zmqpp/message.hpp>
26 FLED::FLED(zmqpp::context &ctx,
const std::string &led_name)
27 : backend_(ctx,
zmqpp::socket_type::req)
29 backend_.connect(
"inproc://" + led_name);
38 msg <<
"ON" << duration.count();
51 return turnOn(std::chrono::milliseconds(duration));
106 bool FLED::blink(std::chrono::milliseconds duration, std::chrono::milliseconds speed)
111 msg <<
"BLINK" << duration.count() << speed.count();
124 return blink(std::chrono::milliseconds(duration),
125 std::chrono::milliseconds(speed));
157 std::string status_str;
163 if (status_str ==
"BLINKING")
165 assert(rep.parts() == 4);
172 rep >> led_state.
speed;
176 assert(status_str ==
"ON" || status_str ==
"OFF");
177 led_state.
value = status_str ==
"ON";
181 assert(rep.parts() == 1);
182 if (status_str ==
"ON")
184 else if (status_str ==
"OFF")
int64_t speed
Set only if st is BLINKING, it represents the speed of blinking.
enum Leosac::Hardware::FLED::State::@0 st
Internal state of the LED.
bool turnOff()
Turn the LED OFF by sending a message to the backend LED impl.
FLED(zmqpp::context &ctx, const std::string &led_name)
#define ASSERT_LOG(cond, msg)
bool isOff()
Similar to isOn().
bool isOn()
Query the value of the GPIO and returns true if the LED is ON.
zmqpp::poller poller_
A poller to not wait for infinity in case something went wrong.
zmqpp::socket backend_
A socket to talk to the backend LED.
bool value
Set only if st is BLINKING : value of the LED (true if ON, false otherwise).
State state()
Return the state of the device.
bool toggle()
Toggle the LED value by sending a message to the backend LED impl.
bool isBlinking()
Returns true is the LED is currently blinking.
zmqpp::socket & backend()
Access the backend socket (which is connect to the LED device) to send command directly.
int64_t duration
Set only if st is BLINKING, it represents the total duration of blinking.
bool turnOn()
Turn the LED ON by sending a message to the backend LED impl.
bool blink()
Make the LED blink.
Provides facade classes to hardware device implementation.