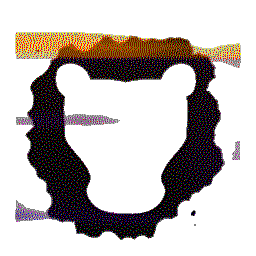 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
30 : sock_(ctx,
zmqpp::socket_type::rep)
32 , gpio_device_(gpio_device)
33 , gpio_offset_(gpio_offset)
34 , direction_(direction)
35 , initial_value_(initial_value)
37 , next_update_time_(std::chrono::system_clock::time_point::max())
38 , gpiod_chip_(nullptr)
39 , gpiod_line_(nullptr)
42 sock_.bind(
"inproc://" + name);
97 ASSERT_LOG(ret >= 0,
"Toggle failed on GPIO pin.");
102 zmqpp::message_t msg;
110 else if (frame1 ==
"OFF")
112 else if (frame1 ==
"TOGGLE")
114 sock_.send(ok ?
"OK" :
"KO");
123 if (msg && msg->remaining() == 1)
131 std::chrono::system_clock::now() + std::chrono::milliseconds(duration);
135 WARN(
"Called with unexpected number of arguments: " << msg->remaining());
151 ASSERT_LOG(ret >= 0,
"Toggle failed on GPIO pin.");
158 ASSERT_LOG(ret >= 0,
"Read failed on GPIO pin.");
164 gpiod_line_event gpiod_event;
165 int ret = gpiod_line_event_read_fd(
gpiod_fd_, &gpiod_event);
166 ASSERT_LOG(ret >= 0,
"Read failed on GPIO pin.");
177 ASSERT_LOG(
gpiod_fd_ >= 0,
"Bad GPIO line or the line is not setup for event monitoring.");
179 zmqpp::poller::poll_pri);
190 DEBUG(
"Turning off Libgpiod pin.");
void set_direction(Direction dir)
Write direction to the direction file.
void handle_message()
The SysFsGpioModule will register this method so its called when a message is ready on the pin socket...
LibgpiodModule & module_
Reference to the module.
void update()
Update the PIN.
#define ASSERT_LOG(cond, msg)
std::chrono::system_clock::time_point next_update() const
This method shall returns the time point at which we want to be updated.
void release()
Release Libgpiod resources.
void publish_on_bus(zmqpp::message &msg)
Write the message eon the bus.
bool turn_on(zmqpp::message *msg=nullptr)
Write to sysfs to turn the gpio on.
Handle GPIO management over libgpiod.
bool toggle()
Read to sysfs and then write the opposite value.
std::shared_ptr< LibgpiodConfig > general_config() const
Retrieve the config object.
std::chrono::system_clock::time_point next_update_time_
Time point of next wished update.
Namespace for the module that implements GPIO support using the Linux Kernel libgpiod interface.
void set_interrupt(InterruptMode mode)
Write interrupt mode to the edge file.
LibgpiodPin(zmqpp::context &ctx, const std::string &name, const std::string &gpio_device, int gpio_offset, Direction direction, InterruptMode interrupt_mode, bool initial_value, LibgpiodModule &module)
void handle_interrupt()
Interrupt happened for this GPIO ping.
void register_sockets(zmqpp::reactor *reactor)
Register own socket to the module's reactor.
const Direction direction_
Direction of the PIN.
bool turn_off()
Write to sysfs to turn the gpio on.
int gpio_offset_
Offset of the GPIO.
std::string gpio_device_
File descriptor of the GPIO in sysfs.
bool read_value()
Read value from filesystem.
const bool initial_value_
Initial value of the PIN.
zmqpp::socket sock_
listen to command from other component.