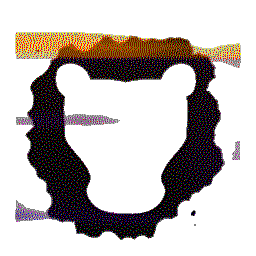 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <boost/property_tree/ptree.hpp>
26 #include <zmqpp/reactor.hpp>
27 #include <zmqpp/socket.hpp>
52 LibgpiodModule(zmqpp::context &ctx, zmqpp::socket *module_manager_pipe,
53 const boost::property_tree::ptree &config,
CoreUtilsPtr utils);
72 virtual void run()
override;
94 std::vector<std::shared_ptr<LibgpiodPin>>
gpios_;
Base class for module implementation.
void process_general_config()
General configuration (file paths, etc).
void process_config(const boost::property_tree::ptree &cfg)
Process the configuration, preparing configured GPIO pin.
zmqpp::socket bus_push_
Socket to write the bus.
LibgpiodModule & operator=(LibgpiodModule &&)=delete
void publish_on_bus(zmqpp::message &msg)
Write the message eon the bus.
This is the header file for a generated source file, GitSHA1.cpp.
Handle GPIO management over libgpiod.
std::shared_ptr< LibgpiodConfig > general_config() const
Retrieve the config object.
LibgpiodModule(zmqpp::context &ctx, zmqpp::socket *module_manager_pipe, const boost::property_tree::ptree &config, CoreUtilsPtr utils)
std::shared_ptr< LibgpiodConfig > general_cfg_
General configuration for module.
virtual void run() override
This is the main loop of the module.
std::vector< std::shared_ptr< LibgpiodPin > > gpios_
Vector of underlying pin object.
std::shared_ptr< CoreUtils > CoreUtilsPtr