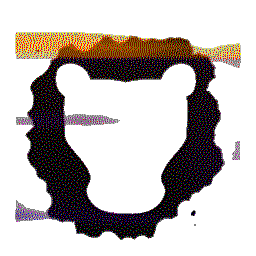 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
27 #include <boost/type_index.hpp>
37 converted_msg.uuid = msg.
uuid;
38 converted_msg.type = msg.
type;
46 static std::string exception_name(
const std::exception_ptr &ptr)
51 std::rethrow_exception(ptr);
54 catch (
const std::exception &e)
56 auto type_index = boost::typeindex::type_id_runtime(e);
57 return type_index.pretty_name();
64 std::string ename = exception_name(ptr);
68 std::rethrow_exception(ptr);
112 WARN(
"Leosac specific exception has been caught: " << e.
what() << std::endl
117 catch (
const odb::exception &e)
119 ERROR(
"Database Error: " << e.what());
121 response.
status_string =
"Database Error: " + std::string(e.what());
123 catch (
const std::exception &e)
125 WARN(
"Exception when processing request: " << e.what());
virtual const char * what() const noexcept final
@ MODEL_EXCEPTION
Some internal API rules has failed.
const Leosac::Tools::Stacktrace & trace() const
Get the stacktrace associated with this exception.
#define BUILD_STR(param)
Internal macro.
APIStatusCode status_code
A message sent by the server to a client.
@ INVALID_ARGUMENT
One of the argument of the call had a invalid value / type.
@ ENTITY_NOT_FOUND
The requested entity cannot be found.
@ SESSION_ABORTED
The session has been aborted.
All modules that provides features to Leosac shall be in this namespace.
ServerMessage convert_merge(const std::exception_ptr &ptr, const ServerMessage &msg)
Convert the exception_ptr to a ServerMessage and merge it with an other message.
@ GENERAL_FAILURE
A failure for an unknown reason.
This is the header file for a generated source file, GitSHA1.cpp.
std::string status_string
@ PERMISSION_DENIED
The websocket connection is not allowed to make the requested API call.
A base class for Leosac specific exception.
A class to represents invalid argument exception in Leosac.
json json_errors() const
Format the ModelError object(s).
@ MALFORMED
The source packet was malformed.
virtual ServerMessage convert_impl(const std::exception_ptr &ptr)
const std::string & entity_type() const
An exception class for general API error.
@ INVALID_CALL
The API method (ie, message's type) does not exist.
@ DATABASE_ERROR
An internal database operation threw an exception.
const std::string & entity_id() const
An exception that can be throw when the permission for a given operation is denied.