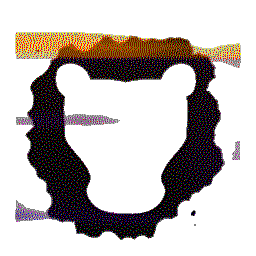 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
21 #include "pifacedigital.h"
25 Direction direction,
bool value, uint8_t hardware_address)
27 , sock_(ctx,
zmqpp::socket_type::rep)
28 , bus_push_(new
zmqpp::socket(ctx,
zmqpp::socket_type::push))
30 , direction_(direction)
31 , default_value_(value)
32 , hardware_address_(hardware_address)
35 DEBUG(
"trying to bind to " << (
"inproc://" + name));
36 sock_.bind(
"inproc://" + name);
37 bus_push_->connect(
"inproc://zmq-bus-pull");
51 : sock_(std::move(o.sock_))
52 , direction_(o.direction_)
53 , default_value_(o.default_value_)
56 this->
name_ = o.name_;
61 o.bus_push_ =
nullptr;
74 else if (frame1 ==
"OFF")
76 else if (frame1 ==
"TOGGLE")
78 else if (frame1 ==
"STATE")
81 ERROR(
"Invalid command received (" << frame1
82 <<
"). Potential missconfiguration !");
83 sock_.send(ok ?
"OK" :
"KO");
91 if (msg && msg->parts() > 1)
97 std::chrono::system_clock::now() + std::chrono::milliseconds(duration);
135 return pifacedigital_read_bit(
150 return std::chrono::system_clock::time_point::max();
PFDigitalPin(zmqpp::context &ctx, const std::string &name, int gpio_no, Direction direction, bool value, uint8_t hardware_address)
Create a new GPIO pin.
std::chrono::system_clock::time_point next_update() const
This method shall returns the time point at which we want to be updated.
This is a implementation class.
const Direction direction_
This is the direction of the GPIO pin.
void update()
Let the GPIO pin perform internal task.
uint8_t hardware_address_
bool turn_on(zmqpp::message *msg=nullptr)
Write to PFDigital to turn the gpio on.
void handle_message()
The PFGpioModule will register this method so its called when a message is ready on the pin socket.
zmqpp::socket * bus_push_
PUSH socket to write to the bus.
void send_state()
Send the current GPIO state on the socket.
bool read_value()
Ask the PiFace device for this pin's value and return it.
bool want_update_
Does this object wants to be update()d ?
bool turn_off()
Write to PFDigital turn the gpio off.
bool default_value_
The default value of the pin, it is only relevant is the pin is output.
std::chrono::system_clock::time_point next_update_time_
Time point of next wished update.
zmqpp::socket sock_
listen to command from other component.