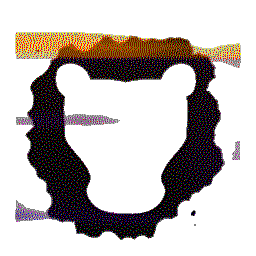 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include <zmqpp/zmqpp.hpp>
47 PFDigitalPin(zmqpp::context &ctx,
const std::string &name,
int gpio_no,
48 Direction direction,
bool value, uint8_t hardware_address);
76 std::chrono::system_clock::time_point
next_update()
const;
83 bool turn_on(zmqpp::message *msg =
nullptr);
PFDigitalPin(zmqpp::context &ctx, const std::string &name, int gpio_no, Direction direction, bool value, uint8_t hardware_address)
Create a new GPIO pin.
std::chrono::system_clock::time_point next_update() const
This method shall returns the time point at which we want to be updated.
PFDigitalPin & operator=(const PFDigitalPin &)=delete
This is a implementation class.
const Direction direction_
This is the direction of the GPIO pin.
void update()
Let the GPIO pin perform internal task.
uint8_t hardware_address_
bool turn_on(zmqpp::message *msg=nullptr)
Write to PFDigital to turn the gpio on.
void handle_message()
The PFGpioModule will register this method so its called when a message is ready on the pin socket.
zmqpp::socket * bus_push_
PUSH socket to write to the bus.
void send_state()
Send the current GPIO state on the socket.
bool read_value()
Ask the PiFace device for this pin's value and return it.
bool want_update_
Does this object wants to be update()d ?
bool turn_off()
Write to PFDigital turn the gpio off.
bool default_value_
The default value of the pin, it is only relevant is the pin is output.
std::chrono::system_clock::time_point next_update_time_
Time point of next wished update.
zmqpp::socket sock_
listen to command from other component.