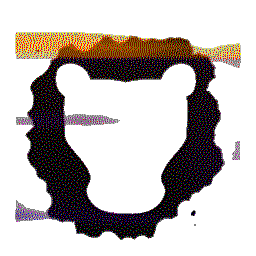 |
Leosac
0.8.0
Open Source Access Control
|
Holds classes relevant to the Authentication and Authorization subsystem.
virtual const ValidityInfo & validity() const override
Get the object that store info about the credential's validity.
std::string id_
Credentials ID.
std::shared_ptr< User > UserPtr
UserPtr owner_
Which user this auth source maps to.
BaseAuthSource(const std::string &id="")
std::shared_ptr< IAccessProfile > IAccessProfilePtr
std::shared_ptr< IAuthenticationSource > IAuthenticationSourcePtr
std::vector< IAuthenticationSourcePtr > subsources_
Underlying auth source.
virtual UserPtr owner() const override
Retrieve the user that map to this source.
std::string source_name_
Name of the source (generally the module / device that sent it)
virtual IAccessProfilePtr profile() const override
This implementation simply return the profile associated with the credential, or null if not availabl...
virtual const std::string & name() const override
Return the name of the source.
virtual const std::string & id() const override
Returns the ID of the credentials.
IAccessProfilePtr profile_
virtual void addAuthSource(IAuthenticationSourcePtr source) override
Adds a new authentication sources as a subsource of this one.
virtual std::string to_string() const override
Returns a string representation of the authentication source content.
A simple class that stores (and can be queried for) the validity of some objects.