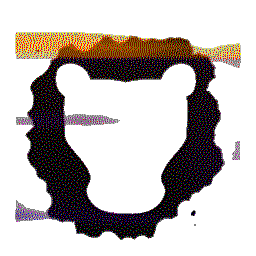 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
55 virtual const std::string &
name()
const override;
60 void name(
const std::string &n);
62 virtual std::string
to_string()
const override;
64 virtual const std::string &
id()
const override;
66 void id(
const std::string &cred_id)
override;
virtual const ValidityInfo & validity() const override
Get the object that store info about the credential's validity.
std::string id_
Credentials ID.
std::shared_ptr< User > UserPtr
UserPtr owner_
Which user this auth source maps to.
BaseAuthSource(const std::string &id="")
std::shared_ptr< IAccessProfile > IAccessProfilePtr
This is the header file for a generated source file, GitSHA1.cpp.
std::shared_ptr< IAuthenticationSource > IAuthenticationSourcePtr
std::vector< IAuthenticationSourcePtr > subsources_
Underlying auth source.
virtual UserPtr owner() const override
Retrieve the user that map to this source.
std::string source_name_
Name of the source (generally the module / device that sent it)
virtual ~BaseAuthSource()=default
virtual IAccessProfilePtr profile() const override
This implementation simply return the profile associated with the credential, or null if not availabl...
An Auth source is a card id, a pin code, a fingerprint, etc...
std::shared_ptr< BaseAuthSource > BaseAuthSourcePtr
virtual const std::string & name() const override
Return the name of the source.
virtual const std::string & id() const override
Returns the ID of the credentials.
IAccessProfilePtr profile_
virtual void addAuthSource(IAuthenticationSourcePtr source) override
Adds a new authentication sources as a subsource of this one.
virtual std::string to_string() const override
Returns a string representation of the authentication source content.
A simple class that stores (and can be queried for) the validity of some objects.