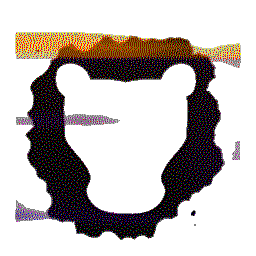 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
42 std::size_t toRead = buffer.
toRead();
50 if (toRead < packet.
dataLen + 4U)
55 for (
unsigned int i = 0; i < packet.
dataLen; ++i)
85 for (
int i = 0; i < packet.
dataLen; ++i)
90 return (packet.
dataLen + 4 + 1);
static const std::size_t CommandByteIdx
static RplethPacket decodeCommand(CircularBuffer &buffer, bool from_server=false)
Decode a packet from a circular buffer object.
static std::size_t encodeCommand(const RplethPacket &packet, Byte *buffer, std::size_t size)
void fastForward(std::size_t offset)
Namespace where implementation for Rpleth support takes place.
static const std::size_t TypeByteIdx
std::size_t toRead() const
Rpleth protocol implementation.
static const std::size_t PacketMinSize
Implementation of a ring buffer.
static const std::size_t SizeByteIdx