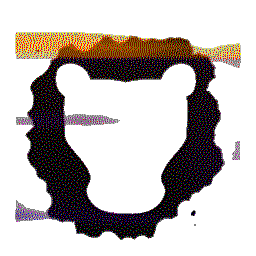 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #ifndef CIRCULARBUFFER_HPP
27 #define CIRCULARBUFFER_HPP
53 std::size_t
read(
Byte *data, std::size_t size);
55 std::size_t
write(
const Byte *data, std::size_t size);
65 std::size_t
toRead()
const;
79 #endif // CIRCULARBUFFER_HPP
CircularBuffer(std::size_t size=DefaultSize)
Byte operator[](int idx) const
std::size_t read(Byte *data, std::size_t size)
~CircularBuffer()=default
This is the header file for a generated source file, GitSHA1.cpp.
void fastForward(std::size_t offset)
std::size_t toRead() const
static const std::size_t DefaultSize
buffer-related helper functions
Implementation of a ring buffer.
std::vector< Byte > _buffer
std::size_t write(const Byte *data, std::size_t size)
std::size_t getSize() const