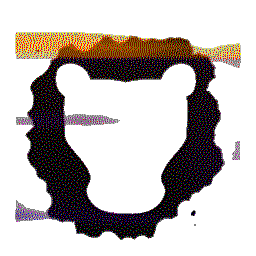 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include "core/audit/ZoneEvent_odb.h"
24 #include "core/auth/Zone_odb.h"
42 ASSERT_LOG(target_zone,
"Target zone must be non null.");
43 ASSERT_LOG(target_zone->id(),
"Target zone must be already persisted.");
52 audit->target(target_zone);
67 auto zone_odb = std::dynamic_pointer_cast<Auth::Zone>(zone);
68 ASSERT_LOG(zone_odb,
"IZone is not of type Zone.");
105 std::stringstream ss;
124 desc[
"alias"] = t->alias();
131 return std::shared_ptr<ZoneEvent>(
new ZoneEvent());
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
void commit()
Commit the transaction, if there was no currently active transaction at the time of this object's cre...
std::shared_ptr< AuditEntry > AuditEntryPtr
Auth::ZoneId target_zone_id_
#define ASSERT_LOG(cond, msg)
void database(DBPtr db)
Set the database pointer.
virtual const std::string & before() const override
std::shared_ptr< odb::database > DBPtr
Provides an implementation of IZoneEvent.
virtual void target(Auth::IZonePtr zone) override
Set the zone that is targeted by the event.
virtual std::string generate_description() const override
Generate a description for this event.
The Audit namespace provides classes and facilities to keep track of what's happening on the Leosac d...
This is the header file for a generated source file, GitSHA1.cpp.
virtual bool finalized() const override
Is this entry finalized.
std::string after_
Optional JSON dump of the object after the event took place.
std::string generate_target_description() const
Generate a short description for the targeted zone.
virtual Auth::ZoneId target_id() const override
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
std::shared_ptr< ZoneEvent > ZoneEventPtr
std::shared_ptr< IZone > IZonePtr
static std::shared_ptr< ZoneEvent > create_empty()
static std::shared_ptr< ZoneEvent > create(const DBPtr &database, Auth::IZonePtr target_zone, AuditEntryPtr parent)
std::string before_
Optional JSON dump of the object before the event took place.
virtual const std::string & after() const override