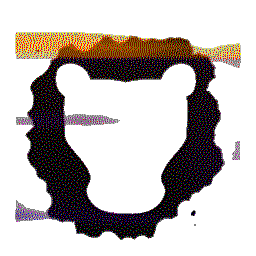 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include "gtest/gtest.h"
42 ctx_ =
new zmqpp::context_t();
56 boost::property_tree::ptree aliases_cfg, module_cfg;
58 module_cfg.add(
"export_path",
"/path/to/export");
59 module_cfg.add(
"unexport_path",
"/path/to/unexport");
60 module_cfg.add(
"value_path",
"/path/to/gpios/__PLACEHOLDER__/value");
61 module_cfg.add(
"edge_path",
"/path/to/gpios/__PLACEHOLDER__/edge");
62 module_cfg.add(
"direction_path",
"/path/to/gpios/__PLACEHOLDER__/direction");
64 module_cfg.add_child(
"aliases", aliases_cfg);
65 cfg_case_1_ = module_cfg;
70 boost::property_tree::ptree aliases_cfg, module_cfg;
72 module_cfg.add(
"export_path",
"/path/to/export");
73 module_cfg.add(
"unexport_path",
"/path/to/unexport");
74 module_cfg.add(
"value_path",
"/random/path/to/__PLACEHOLDER__/value");
75 module_cfg.add(
"edge_path",
"/random/path/to/__PLACEHOLDER__/edge");
76 module_cfg.add(
"direction_path",
77 "/random/path/to/__PLACEHOLDER__/direction");
80 aliases_cfg.add(
"default",
"gpio__NO__");
81 module_cfg.add_child(
"aliases", aliases_cfg);
82 cfg_case_2_ = module_cfg;
87 boost::property_tree::ptree aliases_cfg, module_cfg;
89 module_cfg.add(
"export_path",
"/path/to/export");
90 module_cfg.add(
"unexport_path",
"/path/to/unexport");
91 module_cfg.add(
"value_path",
"/path/to/gpios/__PLACEHOLDER__/value");
92 module_cfg.add(
"edge_path",
"/path/to/gpios/__PLACEHOLDER__/edge");
93 module_cfg.add(
"direction_path",
"/path/to/gpios/__PLACEHOLDER__/direction");
96 aliases_cfg.add(
"default",
"gpio__NO__");
98 aliases_cfg.add(
"21",
"gpio_magic_number");
99 module_cfg.add_child(
"aliases", aliases_cfg);
101 cfg_case_3_ = module_cfg;
114 ASSERT_EQ(
"/path/to/export", my_config.
export_path());
122 ASSERT_EQ(
"/random/path/to/gpio14/value", my_config.
value_path(14));
123 ASSERT_EQ(
"/random/path/to/gpio3/value", my_config.
value_path(3));
124 ASSERT_EQ(
"/random/path/to/gpio17/value", my_config.
value_path(17));
131 ASSERT_EQ(
"/path/to/gpios/gpio14/value", my_config.
value_path(14));
132 ASSERT_EQ(
"/path/to/gpios/gpio3/value", my_config.
value_path(3));
133 ASSERT_EQ(
"/path/to/gpios/gpio_magic_number/value", my_config.
value_path(21));
135 ASSERT_EQ(
"/path/to/gpios/gpio3/edge", my_config.
edge_path(3));
136 ASSERT_EQ(
"/path/to/gpios/gpio_magic_number/edge", my_config.
edge_path(21));
138 ASSERT_EQ(
"/path/to/gpios/gpio3/direction", my_config.
direction_path(3));
139 ASSERT_EQ(
"/path/to/gpios/gpio_magic_number/direction",
std::string direction_path(int pin_no) const
Compute the absolute path the "direction" file for pin_no.
boost::property_tree::ptree cfg_case_1_
void build_config_case2()
Note this class doesnt inherits the TestHelper class.
Internal configuration helper for sysfsgpio module.
std::string value_path(int pin_no) const
Compute the absolute path the "value" file for pin_no.
This is the header file for a generated source file, GitSHA1.cpp.
Namespace for the module that implements GPIO support using the Linux Kernel sysfs interface.
std::string edge_path(int pin_no) const
Compute the absolute path the "edge" file for pin_no.
boost::property_tree::ptree cfg_case_3_
void build_config_case1()
void build_config_case3()
boost::property_tree::ptree cfg_case_2_
TEST_F(SysFsGpioConfigTest, MoreComplexeAliases)
const std::string & export_path() const
Returns the absolute path to the "export" sysfs file.
const std::string & unexport_path() const
Returns the absolute path to the "unexport" sysfs file.