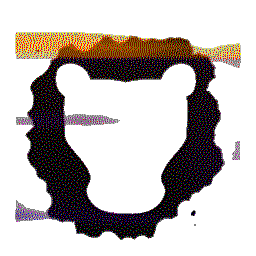 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include "core/auth/Group_odb.h"
26 #include "core/auth/User_odb.h"
28 #include "core/credentials/Credential_odb.h"
29 #include "tools/ScheduleMapping_odb.h"
64 for (
const auto &lazy_weak_user :
users_)
66 if (lazy_weak_user.object_id() == uid)
74 for (
const auto &lazy_weak_group :
groups_)
76 if (lazy_weak_group.object_id() == gid)
84 for (
const auto &lazy_weak_cred :
creds_)
86 if (lazy_weak_cred.object_id() == cid)
94 for (
const auto &lazy_weak_door :
doors_)
96 if (lazy_weak_door.object_id() == did)
109 for (
const auto &group_membership : user->group_memberships())
111 if (
has_group(group_membership->group_id()))
116 for (
const auto &lazy_credentials : user->lazy_credentials())
118 if (
has_cred(lazy_credentials.object_id()))
126 ASSERT_LOG(door,
"Cannot add a null door to a ScheduleMapping.");
129 if (door.get_eager())
130 door->schedule_mapping_added(shared_from_this());
135 ASSERT_LOG(user,
"Cannot add a null user to a ScheduleMapping.");
138 if (user.get_eager())
139 user->schedule_mapping_added(shared_from_this());
144 ASSERT_LOG(group,
"Cannot add a null group to a ScheduleMapping.");
147 if (group.get_eager())
148 group->schedule_mapping_added(shared_from_this());
153 ASSERT_LOG(cred,
"Cannot add a null credential to a ScheduleMapping.");
156 if (cred.get_eager())
157 cred->schedule_mapping_added(shared_from_this());
odb::lazy_shared_ptr< Group > GroupLPtr
#define ASSERT_LOG(cond, msg)
std::shared_ptr< User > UserPtr
This is the header file for a generated source file, GitSHA1.cpp.
odb::lazy_shared_ptr< User > UserLPtr
unsigned long CredentialId
odb::lazy_shared_ptr< Credential > CredentialLPtr
odb::lazy_shared_ptr< Door > DoorLPtr