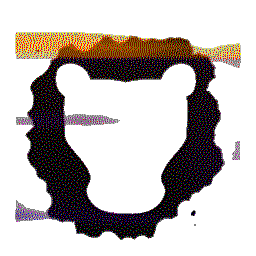 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
47 const boost::property_tree::ptree &cfg,
CoreUtilsPtr utils);
64 static std::vector<uint8_t>
224 std::unique_ptr<Hardware::FWiegandReader>
reader_;
std::list< std::pair< std::string, int > > cards_read_stream_
If stream mode is on, all cards read are stored here.
void rpleth_beep(const RplethPacket &p)
Handle Rpleth Beep command.
Base class for module implementation.
bool push_network_config(const boost::property_tree::ptree &tree)
Push a configuration to the core as the new network config.
void rpleth_greenled(const RplethPacket &p)
Handle rpleth greenled command.
RplethPacket rpleth_receive_cards(const RplethPacket &packet)
Handle Rpleth ReceiveUnpresentedCards command.
simple circular buffer class
void rpleth_publish_card()
Flush the cards_read_stream_ list to clients.
std::unique_ptr< Hardware::FWiegandReader > reader_
Interface to the reader.
RplethPacket set_dhcp_state(const RplethPacket &p)
Enable or disable DHCP.
std::map< std::string, CircularBuffer > clients_
zmqpp::socket server_
Stream socket to receive Rpleth connection.
RplethModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
This is the header file for a generated source file, GitSHA1.cpp.
RplethPacket handle_client_packet(RplethPacket packet)
If we successfully built a packet, lets handle it.
static constexpr int buffer_size
zmqpp::socket bus_sub_
Subscribe to the message bus and listen for event sent by the wiegand reader we watch.
RplethPacket set_reader_ip(const RplethPacket &p)
Update the IP of this Leosac unit.
bool handle_client_msg(const std::string &client_identity, CircularBuffer &buf)
Try to handle a client message.
RplethPacket get_dhcp_state()
Handle Rpleth GetDHCP command.
RplethModule & operator=(const RplethModule &)=delete
zmqpp::socket core_
REQ socket to core.
Main class for the Rpleth module.
static std::vector< uint8_t > card_convert_from_text(const std::pair< std::string, int > &card_info)
Convert a card number from textual hexadecimal representation to a 8 bytes byte-vector in Network Byt...
void rpleth_send_cards(const RplethPacket &packet)
Handle Rpleth SendCards command: we will store the list of received card somewhere safe.
void handle_socket()
Handle data available on server socket.
RplethPacket set_reader_netmask(const RplethPacket &p)
Update netmask of this Leosac unit.
bool client_connected(const std::string &identity) const
Do we already know this client ?
std::list< std::string > cards_read_
Valid cards our Wiegand reader read: cards that were not pushed are not stored here.
void handle_wiegand_event()
We received a message (on the BUS, from the wiegand reader we watch), that means a card was inserted.
Implementation of a ring buffer.
std::shared_ptr< CoreUtils > CoreUtilsPtr
std::vector< std::string > failed_clients_
Client that are "failed".
bool client_failed(const std::string &identity) const
Is the client in an invalid state ?
boost::property_tree::ptree get_network_config()
Retrieve the network configuration from the core.
std::list< std::string > cards_pushed_
List of cards pushed by SendCards Rpleth command.
RplethPacket set_reader_gw(const RplethPacket &p)
Update the reader gateway.