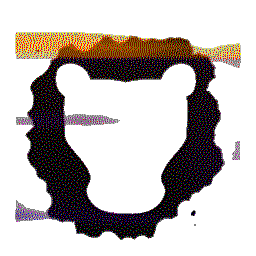 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
21 #include "core/audit/WSAPICall_odb.h"
31 , database_operations_(0)
122 std::stringstream ss;
127 ss <<
"Websocket API call by user " <<
author->username() <<
" to "
132 ss <<
"Websocket API call by anonymous to " <<
method() <<
" took "
virtual void author(Auth::UserPtr user) override
Set the author of the entry.
virtual const std::string & source_endpoint() const override
uint16_t database_operations_
Count the number of database operation that happened while processing this API call.
std::string request_content_
Copy of the JSON content of the request.
static WSAPICallPtr create(const DBPtr &database)
std::string source_endpoint_
The source IP:Port of the client who made the request.
#define ASSERT_LOG(cond, msg)
void database(DBPtr db)
Set the database pointer.
std::shared_ptr< odb::database > DBPtr
std::string status_string_
The status string of the response.
APIStatusCode status_code_
Status code of the response.
virtual const std::string & method() const override
virtual void database_operations(uint16_t nb_operation) override
The number of database queries.
virtual std::string generate_description() const override
Generate a description for this event.
The Audit namespace provides classes and facilities to keep track of what's happening on the Leosac d...
This is the header file for a generated source file, GitSHA1.cpp.
virtual void response_content(const std::string &string) override
virtual const std::string & status_string() const override
size_t duration_
How long did it take for the Audit object to be finalized.
virtual bool finalized() const override
Is this entry finalized.
std::string uuid_
The UUID of the request.
void commit()
Commit the transaction.
virtual const std::string & uuid() const override
Acts like an odb::transaction, with the exception that it will becomes the active transaction at cons...
virtual void request_content(const std::string &string) override
The (JSON) content of the request.
APIStatusCode
Those are the Leosac API status code.
std::shared_ptr< WSAPICall > WSAPICallPtr
virtual APIStatusCode status_code() const override
std::string response_content_
Copy of the JSON content of the response.
Provides the implementation of IWSAPICall.
Auth::UserLPtr author_
The user at the source of the entry.