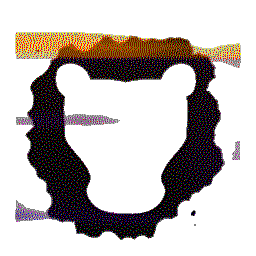 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
21 #include "core/audit/UserEvent_odb.h"
35 ASSERT_LOG(target_user,
"Target user must be non null.");
36 ASSERT_LOG(target_user->id(),
"Target user must be already persisted.");
44 audit->target_ = target_user;
94 using namespace FlagSetOperator;
114 desc[
"username"] = t->username();
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
std::string generate_description() const override
Generate a description for this event.
void commit()
Commit the transaction, if there was no currently active transaction at the time of this object's cre...
std::shared_ptr< UserEvent > UserEventPtr
static std::shared_ptr< UserEvent > create_empty()
std::shared_ptr< AuditEntry > AuditEntryPtr
std::string after_
Optional JSON dump of the object after the event took place.
static std::shared_ptr< UserEvent > create(const DBPtr &database, Auth::UserPtr target_user, AuditEntryPtr parent)
#define ASSERT_LOG(cond, msg)
void database(DBPtr db)
Set the database pointer.
std::shared_ptr< User > UserPtr
const std::string & before() const override
std::shared_ptr< odb::database > DBPtr
Provides an implementation of IUserEvent.
The Audit namespace provides classes and facilities to keep track of what's happening on the Leosac d...
This is the header file for a generated source file, GitSHA1.cpp.
const std::string & after() const override
odb::lazy_shared_ptr< User > UserLPtr
virtual bool finalized() const override
Is this entry finalized.
virtual Auth::UserLPtr target() const override
virtual IAuditEntryPtr parent() const override
Retrieve the parent of this entry.
Auth::UserId target_id() const override
Retrieve the user_id that was targeted by this event.
std::string before_
Optional JSON dump of the object before the event took place.
std::string generate_target_description() const
Generate a small json-string to describe the target user.