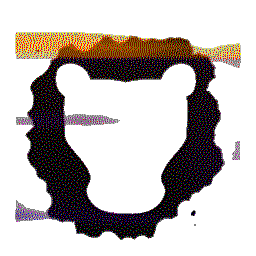 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
76 return door_.lock()->id();
82 ASSERT_LOG(ap_update,
"Cannot attach a null update.");
84 ap_update->access_point(shared_from_this());
Holds classes relevant to the Authentication and Authorization subsystem.
#define ASSERT_LOG(cond, msg)
DoorId door_id() const override
std::shared_ptr< AccessPointUpdate > AccessPointUpdatePtr
std::string controller_module() const override
The name of the module that manages the access point.
This is the header file for a generated source file, GitSHA1.cpp.
std::weak_ptr< Door > door_
std::shared_ptr< IDoor > IDoorPtr
IDoorPtr door() const override
AccessPointId id() const override
const std::string & alias() const override
std::vector< AccessPointUpdateLPtr > updates_
The history of the updates performed against the access-point.
std::string controller_module_
Which module is responsible for this access point.
const std::string & description() const override
void attach_update(AccessPointUpdatePtr)
Attach a new update object to the access-point.
unsigned long AccessPointId