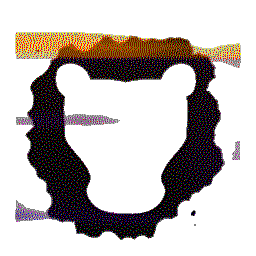 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
65 access_point_->door_ = assert_cast<DoorPtr>(shared_from_this());
std::shared_ptr< AccessPoint > access_point_
The access point that controls the door.
Holds classes relevant to the Authentication and Authorization subsystem.
virtual std::string description() const override
void schedule_mapping_added(const Tools::ScheduleMappingPtr &sched_mapping)
A ScheduleMapping object has added this door as part of its mapping.
virtual AccessPointId access_point_id() const override
std::vector< Tools::ScheduleMappingLWPtr > schedules_mapping_
ScheduleMapping that maps this door.
std::shared_ptr< IAccessPoint > IAccessPointPtr
virtual std::vector< Tools::ScheduleMappingLWPtr > lazy_mapping() const override
Retrieve the lazy pointers to the ScheduleMapping objects that map this door.
virtual DoorId id() const override
virtual std::string alias() const override
virtual IAccessPointPtr access_point() const override
unsigned long AccessPointId