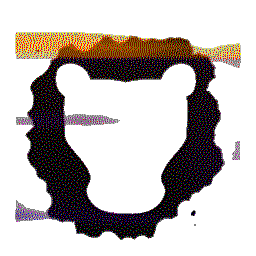 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #include <zmqpp/reactor.hpp>
27 #include <zmqpp/socket.hpp>
78 std::vector<std::string> auth_sources,
79 std::vector<std::string> connect_to,
80 std::vector<std::string> bind_to,
bool act_as_server_
Are we a server or a client ?
void handle_one(zmqpp::message &msg)
std::string zmq_identity_
TargetInfo & operator=(const TargetInfo &o)=default
NotifierInstance & operator=(const NotifierInstance &)=delete
void configure_tcp_socket(const std::vector< std::string > &endpoints)
std::unique_ptr< ProtocolHandler > ProtocolHandlerUPtr
ProtocolHandlerUPtr protocol_
std::unique_ptr< NotifierInstance > NotifierInstanceUPtr
This is the header file for a generated source file, GitSHA1.cpp.
void handle_tcp_msg()
Some event on our ZMQ Stream socket.
zmqpp::socket tcp_
Stream socket used to connect to remote client we want to notify.
~NotifierInstance()=default
TargetInfo * find_target(const std::string &routing_id)
Attempt to find a target from its routing_id.
void handle_credential(Cred::RFIDCard &card)
Notify the peers of the card credential.
std::list< TargetInfo > targets_
NotifierInstance(zmqpp::context &ctx, zmqpp::reactor &reactor, std::vector< std::string > auth_sources, std::vector< std::string > connect_to, std::vector< std::string > bind_to, ProtocolHandlerUPtr protocol_handler)
Create a new notifier instance.
zmqpp::socket bus_sub_
Read internal message bus.
This is an instance of the Notifier.
Some information for each tcp server target.