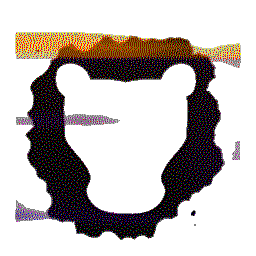 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #include <boost/property_tree/ptree.hpp>
29 #include <zmqpp/zmqpp.hpp>
44 class DoormanInstance;
56 const boost::property_tree::ptree &cfg,
CoreUtilsPtr utils);
64 virtual void run()
override;
66 const std::vector<Auth::AuthTargetPtr> &
doors()
const;
83 std::vector<std::shared_ptr<DoormanInstance>>
doormen_;
88 std::vector<Auth::AuthTargetPtr>
doors_;
Base class for module implementation.
std::vector< std::shared_ptr< DoormanInstance > > doormen_
Authenticator instances.
This is the header file for a generated source file, GitSHA1.cpp.
std::vector< Auth::AuthTargetPtr > doors_
Doors, to manage the always-on or always off stuff.
DoormanModule & operator=(const DoormanModule &)=delete
DoormanModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
virtual void run() override
This is the main loop of the module.
void process_doors_config(const boost::property_tree::ptree &t)
void process_config()
Processing the configuration tree, spawning AuthFileInstance object as described in the configuration...
std::shared_ptr< CoreUtils > CoreUtilsPtr
const std::vector< Auth::AuthTargetPtr > & doors() const
Main class for the module, it create handlers and run them to, well, handle events and send command.