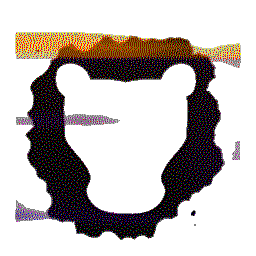 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include <boost/asio/io_service.hpp>
24 #include <boost/asio/steady_timer.hpp>
43 AsioModule(zmqpp::context &ctx, zmqpp::socket *pipe,
44 const boost::property_tree::ptree &cfg,
CoreUtilsPtr utils);
48 virtual void run()
override final;
57 template <
typename Callable>
58 void post(Callable &&callable)
75 std::unique_ptr<boost::asio::io_service::work>
work_;
87 struct StopWatcher :
public std::enable_shared_from_this<StopWatcher>
98 void wait(
const boost::system::error_code &ec);
105 :
public std::enable_shared_from_this<AsyncReactorPoller>
Base class for module implementation.
virtual void on_service_event(const service_event::Event &)=0
Function invoked when a service event is triggered.
virtual void run() override final
This is the main loop of the module.
StopWatcher(AsioModule &self)
bs2::connection service_event_listener_
AsyncReactorPoller(AsioModule &self)
boost::asio::steady_timer timer_
void post(Callable &&callable)
Post some work onto the work queue of the module.
This is the header file for a generated source file, GitSHA1.cpp.
This is a base class for boost::asio 'aware' module.
AsioModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
boost::asio::steady_timer timer_
void wait(const boost::system::error_code &ec)
void wait_handler(const boost::system::error_code &ec)
Poll the zmq reactor from BaseModule.
std::shared_ptr< CoreUtils > CoreUtilsPtr
std::unique_ptr< boost::asio::io_service::work > work_
void install_async_handlers()
Install handlers that periodically poll for activity on the ZMQ reactor from BaseModule.
boost::asio::io_service io_service_