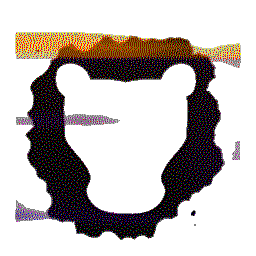 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include "zmqpp/zmqpp.hpp"
24 #include <boost/property_tree/ptree.hpp>
40 class MqttServerConfig;
48 MqttModule(zmqpp::context &ctx, zmqpp::socket *pipe,
49 const boost::property_tree::ptree &cfg,
CoreUtilsPtr utils);
53 virtual void run()
override;
void load_xml_config(const boost::property_tree::ptree &module_config)
Load the module configuration from the XML configuration object.
Base class for module implementation.
virtual void run() override
This is the main loop of the module.
This is the header file for a generated source file, GitSHA1.cpp.
std::vector< MqttExternalServer > servers_
Vector of mqtt connections managed by this module.
void load_db_config()
Load the module configuration from the database.
This simply is the main class for the Wiegand module.
void process_config()
Create Mqtt server instances based on configuration.
std::shared_ptr< CoreUtils > CoreUtilsPtr
MqttModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
std::unique_ptr< MqttConfig > mqtt_config_
Configuration object for the module.